JavaScript events are the cornerstone of interactive web development. They provide a mechanism for your code to respond to user actions, such as clicks, keystrokes, or mouse movements, enabling a dynamic user experience. In this blog, we’ll delve into the basics of JavaScript events, their types, and how to use them effectively in your projects.
What are JavaScript Events?
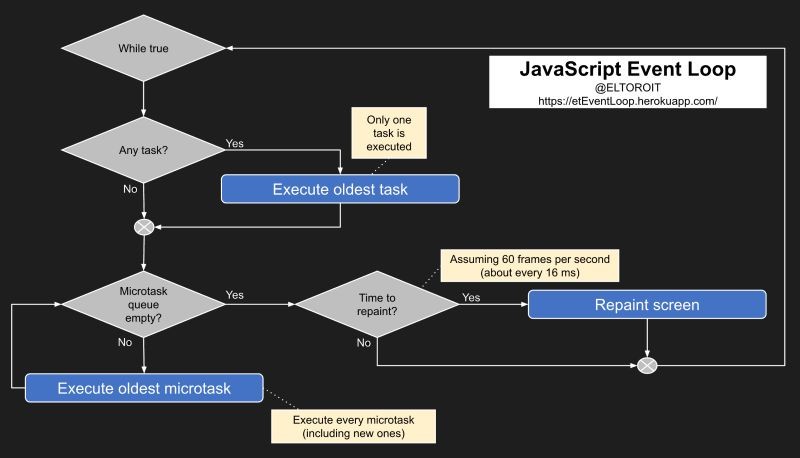
Events in JavaScript are actions or occurrences that happen within the browser. These actions trigger an event, and JavaScript can “listen” for these events to execute specific functions. Whether you’re designing meaningful web pages using HTML semantic elements or enhancing interactivity with JavaScript, mastering event handling is crucial for building user-centric websites.
Types of JavaScript Events
JavaScript events can be broadly categorized as:
- Mouse Events:
click
: Triggered when an element is clicked.dblclick
: Triggered on a double-click.mouseover
: Fires when the pointer is moved over an element.
- Keyboard Events:
keydown
: Fires when a key is pressed.keyup
: Fires when a key is released.
- Form Events:
submit
: Triggered when a form is submitted.change
: Fires when the value of an element changes.
- Window Events:
load
: Fires when the document is fully loaded.resize
: Triggered when the window is resized.
How to Add Event Listeners
JavaScript provides the addEventListener
method to attach event handlers to elements. Here’s an example:
javascriptCopy codeconst button = document.querySelector('#myButton');
button.addEventListener('click', () => {
alert('Button clicked!');
});
Best Practices for Working with Events
- Keep Event Handlers Modular: Avoid writing lengthy inline functions. Instead, define reusable functions.
- Use Delegation for Efficiency: Attach events to a parent element for better performance when working with dynamically added elements.
- Optimize Application Performance: Leverage techniques like caching in full-stack development to reduce unnecessary reflows and repaints caused by frequent DOM updates.
“A well-handled event can make the difference between a basic webpage and a truly interactive experience.” – This philosophy is key to creating intuitive and responsive interfaces.
Advanced Event Handling
For complex web applications, understanding event propagation (bubbling and capturing) is essential. Using tools like CSS preprocessors such as Sass can complement your event handling by enabling better organization of interactive styles.
Real-World Application
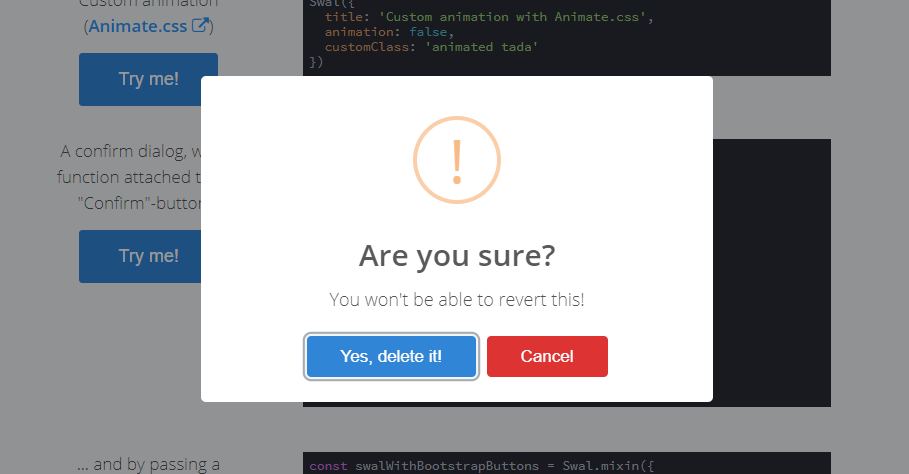
Imagine building a custom dropdown menu:
- Listen for a
click
event to toggle visibility. - Use a
keydown
event to navigate through options with the keyboard. - Attach a
blur
event to close the menu when it loses focus.
By combining these techniques, you can create user-friendly and accessible features.
Conclusion
JavaScript events empower developers to craft engaging and interactive web applications. From basic click events to advanced propagation techniques, mastering events is essential for any front-end developer. Pair these skills with efficient tools and frameworks to build web pages that truly stand out.