JavaScript operators and expressions are essential tools for performing calculations, making decisions, and controlling the behavior of your code. These building blocks are especially important for beginners to understand the fundamentals of programming.
As a developer once said:
“Understanding JavaScript operators is like learning the language’s grammar; it’s what makes your code functional and expressive.”
What Are Operators in JavaScript?
Operators are symbols or keywords that perform operations on data. This data can be variables, constants, or direct values.
Types of Operators in JavaScript:
- Arithmetic Operators:
These operators handle basic mathematical calculations:+
(Addition),-
(Subtraction),*
(Multiplication),/
(Division),%
(Modulus).
let sum = 5 + 10; // Result: 15
- Comparison Operators:
Used to compare values and return true or false:==
(Equal),===
(Strict equal),!=
(Not equal),<
,>
,<=
,>=
.
console.log(10 > 5); // True
- Logical Operators:
Combine multiple conditions:&&
(AND),||
(OR),!
(NOT).
let isLoggedIn = true; let isAdmin = false; console.log(isLoggedIn && isAdmin); // False
- Assignment Operators:
Assign values to variables:=
,+=
,-=
,*=
.
let count = 10; count += 5; // Equivalent to count = count + 5
For more insights on JavaScript fundamentals, check out Mastering JavaScript Variables and Data Types.
Expressions in JavaScript
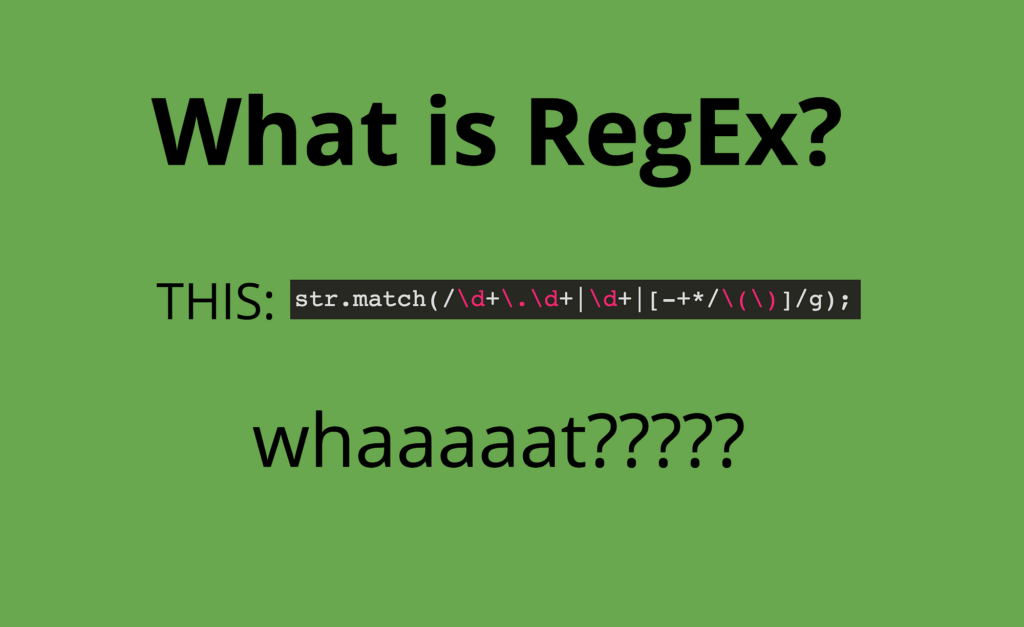
An expression is a piece of code that produces a value. Expressions can involve numbers, strings, or even logical conditions.
- Arithmetic Expressions:javascriptCopy code
let total = (4 + 6) * 3; // Result: 30
- String Expressions:
Combine strings using the+
operator.javascriptCopy codelet greeting = "Hello" + ", " + "world!";
- Logical Expressions:
Use conditions to evaluate values.javascriptCopy codelet canVote = age >= 18 && citizenship === "USA";
- Conditional Expressions:
Use the ternary operator to write conditions concisely.javascriptCopy codelet status = score > 50 ? "Pass" : "Fail";
You can also explore how JavaScript events make websites dynamic by visiting JavaScript Events.
Best Practices for Using Operators and Expressions
- Use Strict Equality:
===
ensures no unintended type conversion. - Parentheses for Clarity: Group expressions for better readability and debugging.javascriptCopy code
let result = (a + b) * c;
- Short-Circuit Logical Operators: Simplify your code with
||
or&&
.javascriptCopy codelet userName = input || "Guest";
To enhance your understanding of website performance and front-end design, read Website Performance Optimization and HTML Semantic Elements: Building Meaningful Web Pages.
Real-World Example
Imagine creating a shopping cart where a discount is applied if the user has a promo code and the total exceeds $100.
javascriptCopy codelet total = 120;
let promoCodeApplied = true;
let finalPrice = promoCodeApplied && total > 100 ? total * 0.9 : total;
console.log(`Final Price: $${finalPrice}`);
This example demonstrates how logical and arithmetic operators work together to build powerful functionalities.
By mastering operators and expressions, you will unlock the potential to write more effective and dynamic JavaScript code. Whether you’re calculating totals, comparing values, or controlling logic flow, these tools are indispensable for building interactive web applications.