JavaScript is the backbone of modern web development, and its vast ecosystem of libraries and frameworks has revolutionized how developers build interactive websites. Among the first and most popular libraries is jQuery, a lightweight, fast, and feature-rich JavaScript library designed to simplify HTML DOM tree traversal, manipulation, event handling, and animation.
In this article, we’ll explore what jQuery is, why it became a game-changer, and how it fits into the broader JavaScript ecosystem. Whether you’re just starting your front-end journey or looking to refresh your skills, understanding jQuery will deepen your knowledge of JavaScript’s evolution and application.
What is jQuery?
jQuery was created in 2006 by John Resig with the goal of simplifying JavaScript’s complexity and making client-side scripting more approachable. Its famous tagline, “Write less, do more,” captures its essence—providing concise syntax to perform complex tasks.
For example, compare this traditional JavaScript code for selecting an element:
javascriptCopy codedocument.getElementById("example").style.color = "blue";
With the jQuery equivalent:
javascriptCopy code$("#example").css("color", "blue");
As seen above, jQuery reduces verbosity and improves code readability.
Why Use jQuery?
- Cross-Browser Compatibility:
Before modern JavaScript engines became uniform, browser inconsistencies caused headaches for developers. jQuery abstracts these differences, providing a unified API across all major browsers. - Easy DOM Manipulation:
Manipulating the DOM with vanilla JavaScript can be tedious. jQuery streamlines these operations using concise selectors and methods. - Event Handling Made Simple:
Handling JavaScript events can be tricky, but jQuery simplifies the process by providing consistent and powerful event methods. For more on JavaScript events, check out this guide: Understanding JavaScript Events.
Getting Started with jQuery
Installation
You can include jQuery in your project by using a CDN or downloading the library:
htmlCopy code<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Alternatively, include it in your package manager using npm:
bashCopy codenpm install jquery
Basic Syntax
The core jQuery function is $()
, which wraps DOM elements and applies various methods. Here’s a basic example:
javascriptCopy code$(document).ready(function() {
$("#button").click(function() {
alert("Button clicked!");
});
});
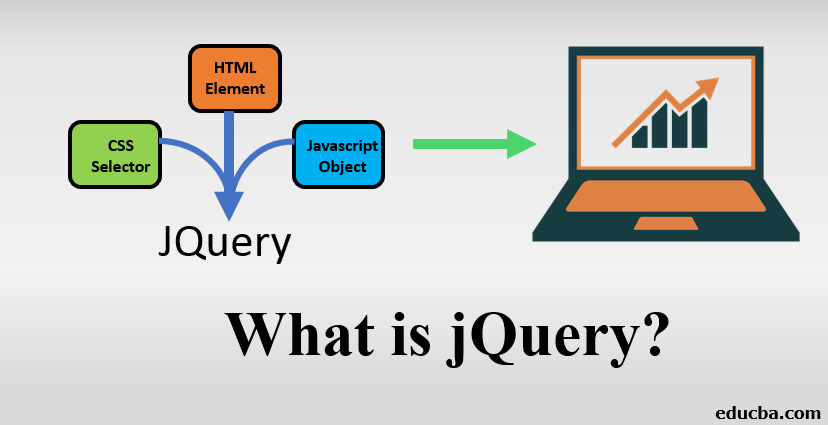
Core Features of jQuery
1. DOM Manipulation
jQuery provides numerous methods to manipulate HTML elements effortlessly:
javascriptCopy code// Changing text
$("#example").text("Hello, World!");
// Adding a class
$("p").addClass("highlight");
// Removing an element
$("#deleteMe").remove();
2. Event Handling
Event handling in jQuery simplifies the way developers interact with user inputs:
javascriptCopy code$("#form").submit(function(event) {
event.preventDefault();
alert("Form submitted!");
});
3. Animations
jQuery includes built-in methods for animations, such as fading, sliding, and custom animations:
javascriptCopy code$("#box").fadeOut(1000);
4. AJAX Support
Asynchronous JavaScript and XML (AJAX) allows web pages to update without reloading. jQuery simplifies AJAX requests:
javascriptCopy code$.get("data.json", function(response) {
console.log(response);
});
The Role of jQuery in Modern Development
Although modern JavaScript frameworks like React and Vue have gained popularity, jQuery remains relevant for quick projects, legacy systems, and cases where simplicity is prioritized over complexity.
Many new developers still use jQuery to grasp core concepts of JavaScript manipulation. In fact, understanding JavaScript libraries like jQuery complements a solid foundation in HTML, CSS, and JavaScript, which are essential for any front-end developer. Learn more about their significance here.
Common Criticisms and Misconceptions
- Is jQuery Obsolete?
While some argue that native JavaScript has caught up, others point out that jQuery remains an efficient solution for many tasks, particularly in environments where older browsers are still in use. - Performance Concerns
Modern JavaScript frameworks often outperform jQuery in large-scale applications. However, for smaller projects, the performance impact is negligible. - Redundant for Small Tasks
For minimal manipulations, using native JavaScript may suffice. However, if you’re dealing with complex animations, AJAX requests, or cross-browser inconsistencies, jQuery shines.
“JavaScript libraries like jQuery serve as stepping stones to mastering core JavaScript, providing an accessible path for developers of all levels.”
How jQuery Enhances JavaScript Mastery
By learning jQuery, developers also deepen their understanding of JavaScript concepts, such as DOM traversal, event handling, and asynchronous programming.
For a deeper dive into JavaScript’s building blocks like variables and data types, explore this insightful article: Mastering JavaScript Variables and Data Types.
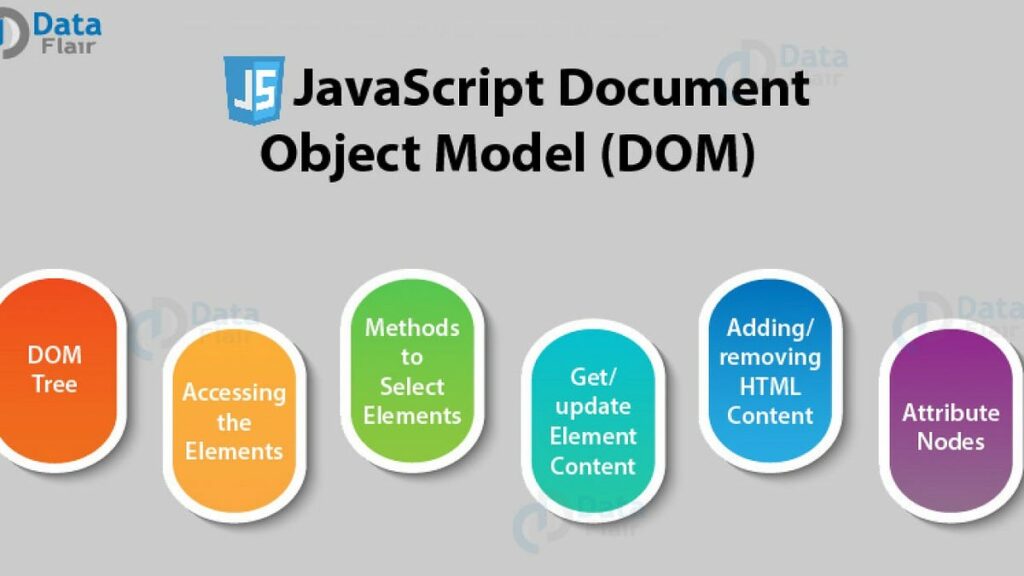
Best Practices for Using jQuery
- Use CDN: Leverage a CDN to deliver jQuery, ensuring faster load times and caching benefits.
- Minimize jQuery Dependence: Use it for tasks that benefit from its concise syntax, while avoiding over-reliance when native JavaScript is more appropriate.
- Follow Modern Standards: Combine jQuery with modern ES6 features for cleaner, more efficient code.
Conclusion
jQuery continues to be a versatile tool in the front-end developer’s toolkit. Whether you’re building quick prototypes or managing legacy projects, its ease of use, cross-browser compatibility, and rich feature set make it invaluable.
By incorporating jQuery into your projects, you’re not only simplifying your workflow but also reinforcing essential JavaScript concepts that are vital for modern web development.
Explore related resources to deepen your understanding of JavaScript libraries and frameworks:
- Why HTML, CSS, and JavaScript are Essential for Front-End Developers
- Understanding JavaScript Events
- Mastering JavaScript Variables and Data Types
Start your jQuery journey today and watch your web development skills reach new heights!