In full-stack development, managing state is one of the core challenges when building dynamic, data-driven applications. State refers to the data that reflects the current status of an application, and as applications grow more complex, managing state consistently across different layers—front-end, back-end, and database—becomes crucial.
For full-stack developers, mastering state management helps ensure data consistency, enhances user experience, and improves app performance. In this post, we’ll dive into state management principles, best practices, and popular tools, all of which are essential for building robust full-stack applications.
What is Application State?
State represents any information that changes over time and influences an application’s behavior. This can include user interactions, real-time data updates, and the app’s responses to server requests. In a typical full-stack application, state flows between multiple layers, including:
- Client-Side State: Data that is temporarily stored in the browser or front-end application to manage interactions and user experiences.
- Server-Side State: Information stored on the server, often sent to the client through APIs and requiring synchronization with the front end.
- Persistent State: Long-term data stored in a database, representing the foundational information (e.g., user profiles, settings) that an application relies on for consistency.
In a simple application, state management may seem straightforward. However, in a full-stack context where different components interact in real-time, state management is challenging but essential to maintain.
Importance of Effective State Management for Full-Stack Developers
Full-stack developers require a broad set of skills, including state management proficiency, to handle complex data flows and ensure consistency across an app’s front and back ends. Building this expertise means understanding the essentials, from client-side storage to server-side data handling. Mastering these essential skills provides developers with the tools to manage state effectively across different layers.
Key Approaches to State Management in Full Stack Applications
Below are the main approaches developers use to manage state, each of which serves unique purposes depending on the application’s requirements:
1. Client-Side State Management
Client-side state management involves data that is used solely within the front-end environment. It handles immediate user interactions, navigation, and session data that doesn’t need to be saved permanently. Popular tools for client-side state management include:
- Redux: A predictable state container for JavaScript apps, particularly useful in React. Redux centralizes state, making it easier to debug and manage across components.
- React Context API: Built into React, Context API allows state to be shared without passing props down manually through the component tree.
- MobX: An alternative to Redux, MobX emphasizes reactivity, making it efficient for automatically syncing state across the application as data changes.
While these tools manage state locally within the client-side environment, for applications that require real-time data or synchronization across multiple users, client-side state must communicate with the server for updates.
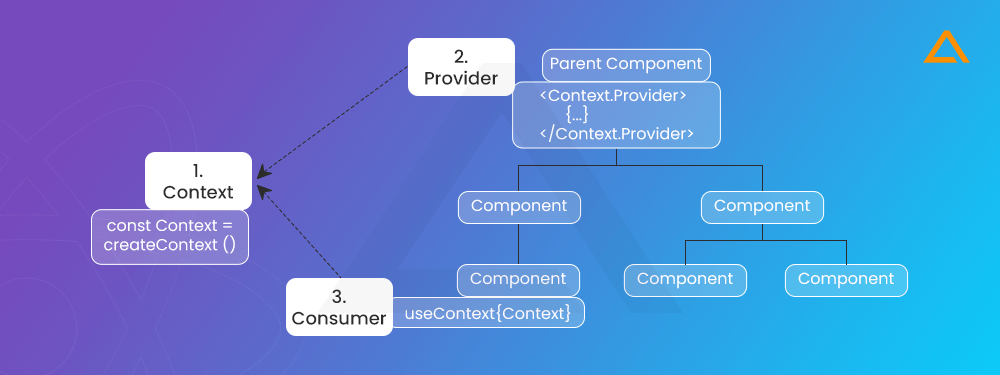
2. Server-Side State Management
Server-side state management deals with state that needs to persist beyond a single session and often requires syncing between multiple clients. The back end typically stores this information and shares it with clients via APIs. Common server-side state management methods include:
- RESTful APIs: Allow the client to fetch or update state on the server through HTTP requests. RESTful design helps manage resources consistently and securely.
- GraphQL: Enables clients to request only the data they need, which is efficient for reducing load times and optimizing server interactions.
- WebSockets: For real-time applications, WebSockets provide continuous, bidirectional communication between the client and server. This is ideal for applications that require frequent updates, like chat apps and online gaming.
Server-side state requires careful design to avoid data conflicts and ensure security. Full-stack developers who have mastered these essential skills know how to implement server-side state efficiently, which enhances the app’s scalability and responsiveness.
Quote: “Effective state management is the backbone of a scalable and responsive application, empowering developers to build dynamic experiences for users.”
3. Persistent State Management in Databases
Persistent state refers to data stored permanently in a database. This data remains available even if the app restarts or experiences interruptions, ensuring a seamless user experience. Database management tools commonly used in persistent state management include:
- MongoDB: A NoSQL database that stores data in a flexible JSON format, making it ideal for applications with evolving data requirements.
- PostgreSQL: An open-source relational database known for robustness and supporting complex queries.
- Redis: Often used as a caching layer for frequently requested data, Redis enhances performance by temporarily storing state that clients regularly access.
To manage persistent state, developers rely on databases that can handle a high volume of transactions, which helps maintain application stability and optimize response times. For teams evaluating a full-stack developer’s capabilities, understanding their ability to manage persistent data is crucial. If you’re looking to hire the right fit, this guide on evaluating full-stack developer ratings offers helpful insights.
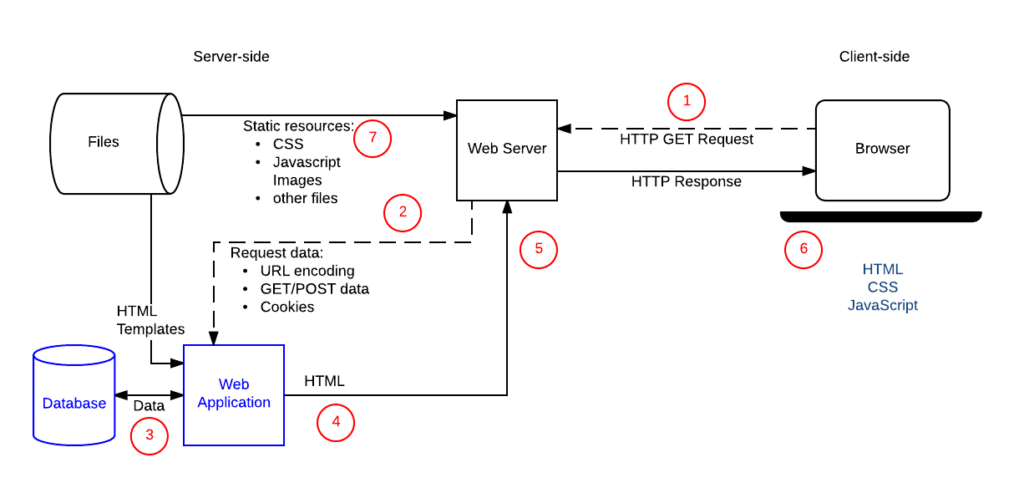
Popular Tools and Libraries for State Management
A range of tools exists for developers to manage state effectively across different environments:
- Redux: Redux’s global state container helps manage complex data flow in React applications. It is particularly beneficial for managing states in larger applications where multiple components need access to the same data.
- MobX: Known for its simplicity and reactivity, MobX automatically tracks data changes, making it efficient for applications where state needs constant syncing across multiple components.
- Apollo Client: A comprehensive state management solution for GraphQL-based applications that enables developers to combine client-side and server-side state efficiently.
- Firebase Realtime Database: Firebase offers real-time synchronization, ideal for applications that need state management without complex back-end setup.
- Redis: Often used for temporary state management and caching, Redis is fast and efficient for frequently accessed data.
These tools offer full-stack developers versatile options for managing different types of state in an application. Choosing the right one depends on the project’s needs and the technical requirements of the development team.
Best Practices for Managing State in Full Stack Applications
- Keep State Close to Where It’s Used: Minimize prop drilling by utilizing tools like Context API or component-level state management to keep state local to where it’s needed.
- Separate UI State from Server State: Distinguish between transient UI state (like form input values) and server state (data stored in the database).
- Use Caching for Frequently Accessed Data: Implement caching tools, such as Redis, to improve load times by temporarily storing frequently requested data.
- Handle Asynchronous State Carefully: Use promises or async/await patterns in JavaScript, and avoid making state changes before responses are received to prevent unexpected behavior.
- Optimize State Synchronization Between Front-End and Back-End: In a real-time application, use tools like WebSockets or Firebase to keep the front-end and back-end states aligned for a seamless user experience.
Conclusion
Mastering state management is a critical skill for full-stack developers. Whether it’s managing client-side interactions, ensuring server-side state consistency, or handling persistent data, a comprehensive understanding of state management techniques and tools is essential for building robust, scalable applications. Developers who can effectively implement these principles enhance the user experience, maintain data integrity, and improve application performance across all layers of the stack.