JavaScript is one of the most widely-used programming languages, powering websites, enhancing user experiences, and enabling dynamic interactions. Whether you’re a beginner or an experienced developer, understanding the basics of JavaScript syntax and its core fundamentals is crucial. In this article, we’ll dive into the essentials, laying a strong foundation for your JavaScript journey.
1. What is JavaScript?
JavaScript is a high-level, interpreted scripting language that allows developers to create interactive elements on websites. Unlike HTML and CSS, which structure and style web pages, JavaScript adds dynamic behavior, making your websites more engaging. For instance, when you click a button and see an alert pop up, that’s JavaScript in action!
Quote: “Any application that can be written in JavaScript, will eventually be written in JavaScript.” — Jeff Atwood
2. JavaScript Syntax: The Basics
JavaScript syntax refers to the set of rules that define a correctly structured JavaScript program. Here are some of the core components:
- Variables: Used to store data values. JavaScript uses
var
,let
, andconst
to declare variables.javascriptCopy codelet message = "Hello, World!"; console.log(message);
- Data Types: JavaScript supports multiple data types, including strings, numbers, booleans, objects, and arrays.
- Operators: JavaScript includes arithmetic, comparison, and logical operators for performing calculations and making decisions.javascriptCopy code
let sum = 5 + 3; let isEqual = (sum === 8);
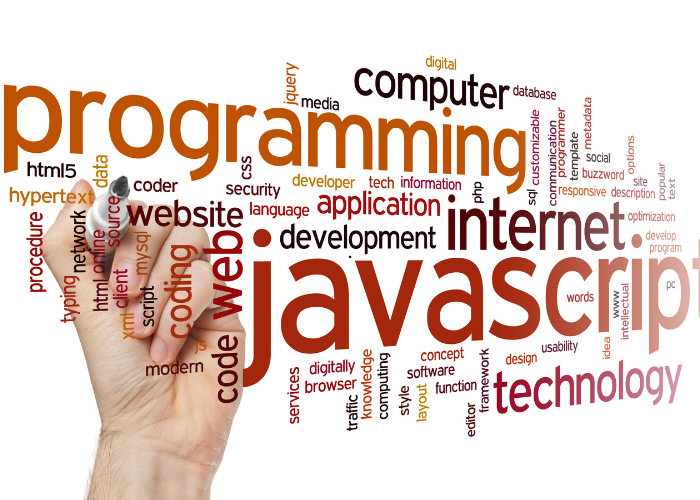
3. JavaScript Fundamentals
Understanding these core concepts will help you build a strong foundation in JavaScript:
- Functions: Reusable blocks of code that perform a specific task. Functions can take parameters and return values.javascriptCopy code
function greet(name) { return `Hello, ${name}!`; } console.log(greet("Alice"));
- Conditionals: JavaScript uses
if
,else
, andswitch
statements to make decisions based on conditions. - Loops:
for
,while
, anddo-while
loops allow you to execute a block of code repeatedly.
4. Introduction to JavaScript Events
Events are actions or occurrences that happen in the browser, like a click or a key press. JavaScript provides powerful ways to handle these events, making your web applications interactive. For a more detailed explanation, check out this comprehensive guide to event handling in JavaScript.
Additionally, mastering events is a key skill for developers. If you want to dive deeper, this comprehensive guide on mastering JavaScript events will give you advanced insights.
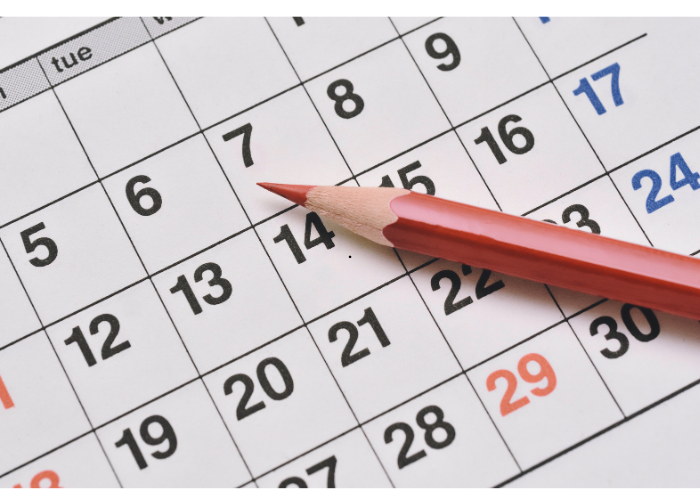
5. Testing in JavaScript
Testing is an essential part of modern JavaScript development, ensuring your code runs as expected. Frameworks like Jest and Mocha simplify the testing process, making it easier to catch errors before they affect users. To learn more, read this introduction to testing in JavaScript using Jest and Mocha.
6. Conclusion
JavaScript is a versatile language that forms the backbone of modern web development. By mastering its syntax and core fundamentals, you set yourself up for success in building dynamic and interactive applications. With a solid grasp of the basics, you’re now ready to explore more advanced topics and enhance your skills.