JavaScript has come a long way since its inception. The release of ECMAScript 2015 (ES6) marked a turning point with new features that streamlined coding and enhanced the functionality of JavaScript. Since then, several new versions (ES7, ES8, and beyond) have introduced even more powerful capabilities. If you want to level up your JavaScript skills, understanding ES6+ features is essential. In this guide, we’ll dive into the key ES6+ features every developer should know.
Why ES6+ is Important for Modern JavaScript Development
The ES6 update brought a host of new features designed to make JavaScript more efficient and readable. These enhancements have become the backbone of modern JavaScript frameworks like React, Angular, and Vue.js. Whether you are building web applications or server-side code with Node.js, leveraging ES6+ features can simplify your code and reduce common pitfalls.
“JavaScript’s evolution with ES6+ has transformed it from a quirky scripting language into a robust, versatile tool for developers.”
Key Features Introduced in ES6
1. Let and Const: Block-Scoped Variables
Before ES6, JavaScript only had function-scoped variables using var
. With the introduction of let
and const
, developers now have better control over variable scope.
let
: Allows block-scoped variables, which means they are limited to the block in which they are declared.const
: For constants that cannot be reassigned once initialized.
Example:
javascriptCopy codelet count = 10;
const maxLimit = 100;
if (count < maxLimit) {
let message = "Count is within the limit.";
console.log(message); // Output: Count is within the limit.
}
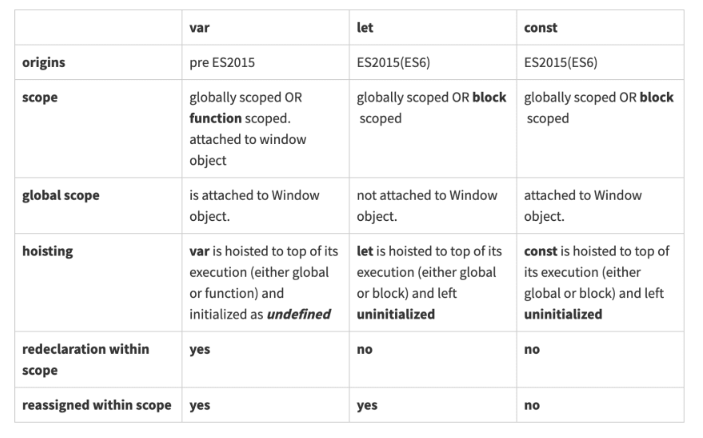
2. Arrow Functions: Shorter Syntax for Functions
Arrow functions provide a more concise syntax for writing functions and also handle the context (this
) differently compared to regular functions.
Example:
javascriptCopy codeconst add = (a, b) => a + b;
console.log(add(5, 10)); // Output: 15
Arrow functions are especially useful for callbacks and array methods like map()
, filter()
, and reduce()
.
3. Template Literals: Enhanced String Interpolation
With template literals, you can embed variables directly into strings using backticks () and
${}` syntax.
Example:
javascriptCopy codeconst name = "Alice";
const greeting = `Hello, ${name}! Welcome to ES6 features.`;
console.log(greeting); // Output: Hello, Alice! Welcome to ES6 features.
This makes constructing strings easier and more readable, especially when dealing with multi-line strings.
4. Default Parameters: Simplifying Function Arguments
Default parameters allow you to set default values for function arguments, reducing the need for additional checks.
Example:
javascriptCopy codefunction greet(name = "Guest") {
return `Hello, ${name}!`;
}
console.log(greet()); // Output: Hello, Guest!
console.log(greet("John")); // Output: Hello, John!
Advanced ES6+ Features: Beyond the Basics
5. Destructuring: Simplifying Object and Array Access
Destructuring lets you unpack values from arrays or properties from objects into distinct variables.
Example:
javascriptCopy codeconst user = { username: "jdoe", age: 30 };
const { username, age } = user;
console.log(username); // Output: jdoe
console.log(age); // Output: 30
6. Spread and Rest Operators: Expanding and Gathering Elements
The spread operator (...
) expands elements of an array, while the rest operator gathers elements into an array.
Example:
javascriptCopy codeconst arr = [1, 2, 3];
const newArr = [...arr, 4, 5];
console.log(newArr); // Output: [1, 2, 3, 4, 5]
function sum(...numbers) {
return numbers.reduce((acc, curr) => acc + curr, 0);
}
console.log(sum(1, 2, 3)); // Output: 6
7. Promises: Asynchronous Programming Made Easy
Promises provide a way to handle asynchronous operations, making code more readable than using callbacks.
Example:
javascriptCopy codeconst fetchData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => resolve("Data fetched successfully"), 1000);
});
};
fetchData().then(data => console.log(data)).catch(error => console.error(error));
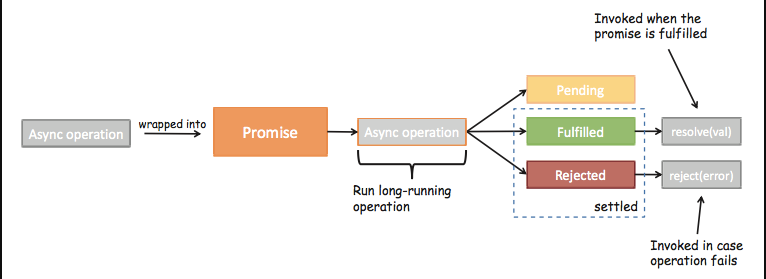
8. Modules: Import and Export
ES6 introduced a standardized module system, allowing developers to export functions, objects, or variables from one file and import them into another.
Example:
javascriptCopy code// math.js
export const multiply = (a, b) => a * b;
// main.js
import { multiply } from './math.js';
console.log(multiply(2, 3)); // Output: 6
ES6+ Features in Action: Leveraging New Tools for Better Code
By integrating ES6+ features into your projects, you can write cleaner, more efficient, and more maintainable code. Frameworks like React and Vue heavily rely on these features to enable powerful and dynamic web applications. For instance, React’s use of hooks like useState
and useEffect
leverages the power of destructuring and arrow functions.
For more insights, you can explore how these features work in tandem with modern frameworks in this comprehensive guide on React Hooks: useState and useEffect.
Embrace the Power of Modern JavaScript
ES6+ has significantly enhanced JavaScript, making it a more powerful and developer-friendly language. By mastering these features, you can write better, cleaner, and more efficient code. Whether you’re building a small script or a large-scale application, embracing ES6+ will help you stay ahead in the evolving world of JavaScript development.
“The future of JavaScript lies in its continual evolution, and ES6+ features are paving the way for more streamlined and efficient code.”
For additional learning, check out this guide on Event Handling in JavaScript and dive deeper into the comparison between GraphQL and REST in this insightful article: GraphQL vs REST.