In the ever-evolving world of web development, choosing the right JavaScript framework or library is crucial for building modern, dynamic applications. Frameworks and libraries like React, Angular, and Vue have become fundamental tools for developers, offering efficient ways to create interactive and scalable user interfaces. In this post, we’ll dive deep into these three popular JavaScript solutions, explore their features, and help you decide which one is best suited for your next project.
Table of Contents
- What Are JavaScript Frameworks and Libraries?
- Overview of React
- Overview of Angular
- Overview of Vue
- Key Differences Between React, Angular, and Vue
- Choosing the Right Framework or Library
- Real-World Use Cases
- Best Practices When Using Frameworks and Libraries
- Conclusion
1. What Are JavaScript Frameworks and Libraries?
JavaScript frameworks and libraries are tools that simplify the development process by providing pre-written code, reusable components, and structured approaches to building web applications. While frameworks like Angular offer a complete solution for building large-scale applications, libraries like React focus on specific parts of the application, such as the user interface.
“The right tool can make or break a project, and in the realm of JavaScript, frameworks and libraries are those essential tools.” — Addy Osmani
Before diving into each tool, it’s helpful to understand the difference:
- Framework: A comprehensive structure that provides a set of guidelines, tools, and best practices (e.g., Angular).
- Library: A collection of functions and utilities focused on solving specific problems (e.g., React, Vue).
Check out this guide for a better understanding of CSS frameworks: Introduction to CSS Frameworks (e.g., Bootstrap).
2. Overview of React
React is a JavaScript library developed by Facebook for building user interfaces, especially single-page applications. React focuses on the View layer of the MVC (Model-View-Controller) architecture, making it flexible and ideal for building dynamic and interactive UI components.
Key Features:
- Component-Based Architecture: React allows you to create reusable components, making your code modular and easier to maintain.
- Virtual DOM: The virtual DOM improves performance by only updating the parts of the DOM that have changed.
- JSX Syntax: React uses JSX, a syntax extension that allows you to write HTML directly within JavaScript.
Example Code:
javascriptCopy codefunction Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
ReactDOM.render(<Welcome name="React" />, document.getElementById('root'));
For more on integrating APIs in React, see this guide: Working with APIs and Fetch in JavaScript.
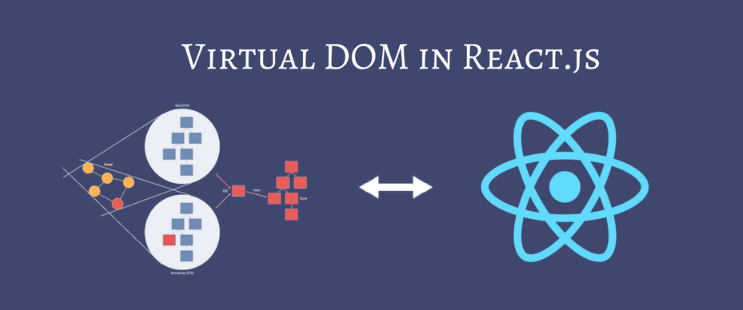
3. Overview of Angular
Angular is a comprehensive JavaScript framework maintained by Google, designed for building complex and robust single-page applications (SPAs). It offers a complete solution with a strong focus on performance and scalability.
Key Features:
- Two-Way Data Binding: Angular automatically synchronizes data between the model and the view, reducing boilerplate code.
- Dependency Injection: Angular’s built-in dependency injection system improves code modularity and testability.
- TypeScript Integration: Angular uses TypeScript, a statically typed superset of JavaScript, enhancing code quality and readability.
Example Code:
typescriptCopy codeimport { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '<h1>Welcome to Angular!</h1>',
})
export class AppComponent {}
Angular is ideal for building enterprise-level applications with complex structures and high scalability needs.
To learn more about handling errors in backend applications, visit: Error Handling in Backend Applications: Best Practices and Techniques.
4. Overview of Vue
Vue is a progressive JavaScript framework designed for building user interfaces. It is often described as a combination of the best features from both React and Angular, offering a lightweight yet powerful solution for front-end development.
Key Features:
- Reactive Data Binding: Vue’s reactivity system ensures that changes in data are automatically reflected in the view.
- Single-File Components: Vue supports single-file components that contain HTML, JavaScript, and CSS, making development streamlined and organized.
- Ease of Integration: Vue is versatile and can be integrated into existing projects incrementally.
Example Code:
vueCopy code<template>
<h1>{{ message }}</h1>
</template>
<script>
export default {
data() {
return {
message: 'Hello, Vue!'
};
}
};
</script>
Vue is well-suited for both small projects and large-scale applications due to its flexibility and ease of use.
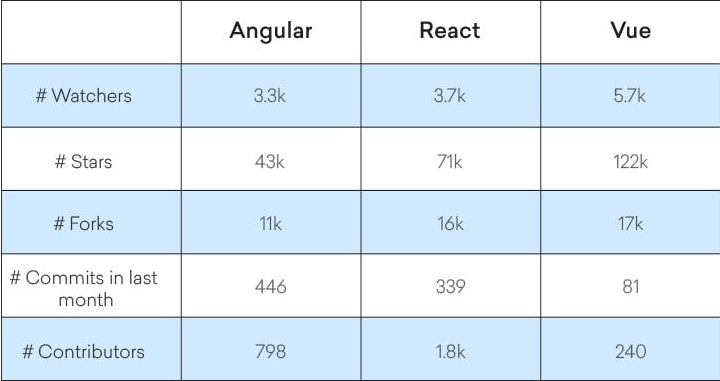
5. Key Differences Between React, Angular, and Vue
Feature | React | Angular | Vue |
---|---|---|---|
Type | Library | Framework | Framework |
Language | JavaScript/JSX | TypeScript | JavaScript/HTML |
Data Binding | One-way | Two-way | Two-way |
Learning Curve | Moderate | Steep | Easy |
Use Cases | Dynamic UI | Enterprise Applications | Versatile Front-End |
“Choosing a framework or library is less about the tool itself and more about the needs of your project.” — Evan You
For a better understanding of JavaScript fundamentals, refer to this post: Introduction to JavaScript Syntax and Fundamentals.
6. Choosing the Right Framework or Library
When deciding which framework or library to use, consider the following:
- Project Requirements: Angular is excellent for large, complex applications; React is best for dynamic UIs; Vue offers a balanced approach for versatile projects.
- Team Expertise: Choose a tool that matches the skill set of your development team.
- Community Support: All three have strong community support, but React’s ecosystem is the largest.
7. Real-World Use Cases
- React: Facebook, Instagram, Airbnb
- Angular: Google Cloud Console, Microsoft Office, Upwork
- Vue: Alibaba, Xiaomi, GitLab
These examples highlight the versatility and scalability of each tool in real-world scenarios.
8. Best Practices When Using Frameworks and Libraries
- Keep Components Small: Write small, reusable components to simplify maintenance.
- Use State Management: Implement state management solutions like Redux (React) or Vuex (Vue) for better data handling.
- Optimize Performance: Use lazy loading, virtual DOM, and efficient rendering techniques to enhance performance.
For more on handling APIs in JavaScript projects, check this guide: Working with APIs and Fetch in JavaScript.
9. Conclusion
React, Angular, and Vue each offer unique strengths, and choosing the right one depends on your project’s needs, your team’s expertise, and your desired learning curve. By understanding the core features and differences of these tools, you can make an informed decision and build efficient, scalable applications.
“Frameworks and libraries are the foundation of modern web development; they allow us to build complex applications quickly and efficiently.” — Brendan Eich
These insights will guide you in selecting the right tool and mastering the art of front-end development. Happy coding!