REST APIs are the backbone of modern web applications, allowing clients to communicate seamlessly with servers. Whether you’re building a simple blog, an e-commerce platform, or a social network, understanding how to create a REST API is essential. This guide will walk you through building a basic REST API using Node.js and Express.js. We’ll cover the setup, defining routes, handling requests, and integrating basic CRUD operations. By the end, you’ll have a functional API ready for further enhancements, such as authentication or database integration.
1. Setting Up Your Node.js Project
Before diving into coding, let’s set up the project environment. You need to have Node.js and npm (Node Package Manager) installed on your machine.
Steps:
- Initialize Your Project: Create a project folder and navigate into it using the terminal. Then, run the following command to initialize a new Node.js project:bashCopy code
mkdir basic-rest-api cd basic-rest-api npm init -y
- Install Dependencies: We need to install Express.js, a minimal and flexible Node.js framework for building APIs.bashCopy code
npm install express
2. Creating the Express Server
Now that we have our project set up, let’s create a basic Express server.
File Structure:
textCopy codebasic-rest-api/
├── node_modules/
├── package.json
└── index.js
Code for index.js
:
javascriptCopy codeconst express = require('express');
const app = express();
const PORT = 3000;
app.use(express.json());
app.get('/', (req, res) => {
res.send('Welcome to our Basic REST API!');
});
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
Run the server with:
bashCopy codenode index.js
Visit http://localhost:3000 in your browser, and you should see a message saying “Welcome to our Basic REST API!”
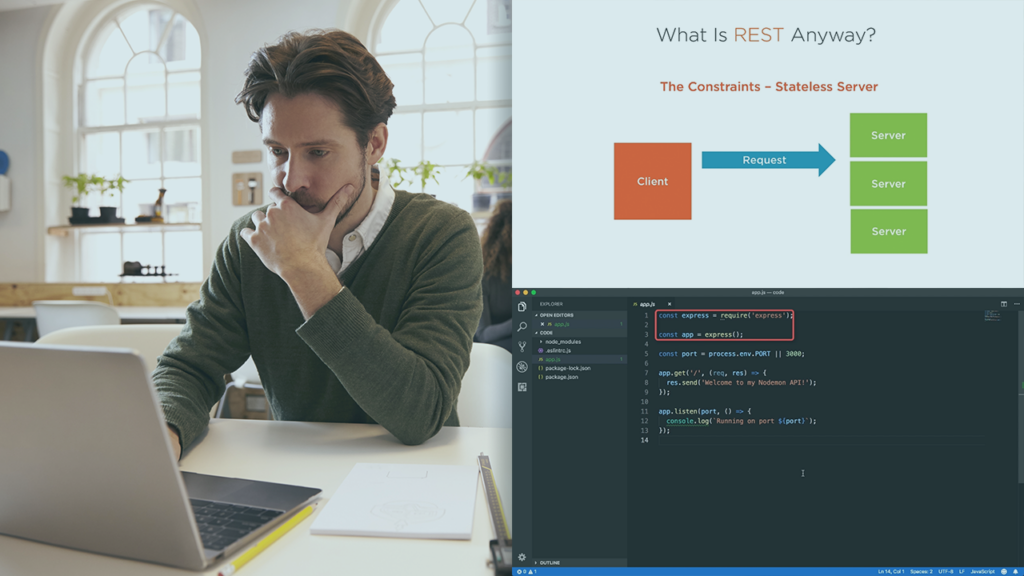
3. Defining RESTful Routes
In REST API design, we use specific HTTP methods like GET
, POST
, PUT
, and DELETE
to handle requests for various resources.
Creating a Simple In-Memory Data Store
For demonstration purposes, we’ll use an array to store data. Here’s a basic example:
javascriptCopy codelet users = [
{ id: 1, name: "John Doe", email: "[email protected]" },
{ id: 2, name: "Jane Doe", email: "[email protected]" }
];
4. Implementing CRUD Operations
We’ll now implement the core CRUD (Create, Read, Update, Delete) operations.
a) GET All Users
javascriptCopy codeapp.get('/users', (req, res) => {
res.json(users);
});
b) GET a Single User by ID
javascriptCopy codeapp.get('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
res.json(user);
});
c) POST – Add a New User
javascriptCopy codeapp.post('/users', (req, res) => {
const newUser = {
id: users.length + 1,
name: req.body.name,
email: req.body.email
};
users.push(newUser);
res.status(201).json(newUser);
});
First Image Placement: This would be an ideal place to include a visual representation of the API’s basic architecture, showing the client-server communication flow for each CRUD operation.
d) PUT – Update an Existing User
javascriptCopy codeapp.put('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
user.name = req.body.name || user.name;
user.email = req.body.email || user.email;
res.json(user);
});
e) DELETE – Remove a User
javascriptCopy codeapp.delete('/users/:id', (req, res) => {
const userIndex = users.findIndex(u => u.id === parseInt(req.params.id));
if (userIndex === -1) return res.status(404).send('User not found');
const deletedUser = users.splice(userIndex, 1);
res.json(deletedUser);
});
5. Testing the API
You can use tools like Postman or cURL to test your API endpoints. Here’s an example of testing the POST request using cURL:
bashCopy codecurl -X POST http://localhost:3000/users -H "Content-Type: application/json" -d '{"name": "Alice", "email": "[email protected]"}'
6. Enhancing Your API with Database Integration
While our current API uses an in-memory data store, in a real-world scenario, you’d likely use a database like MongoDB. If you’re interested in integrating MongoDB with Express, check out this guide on building a simple API with MongoDB and Express and this detailed Medium post on the same topic.
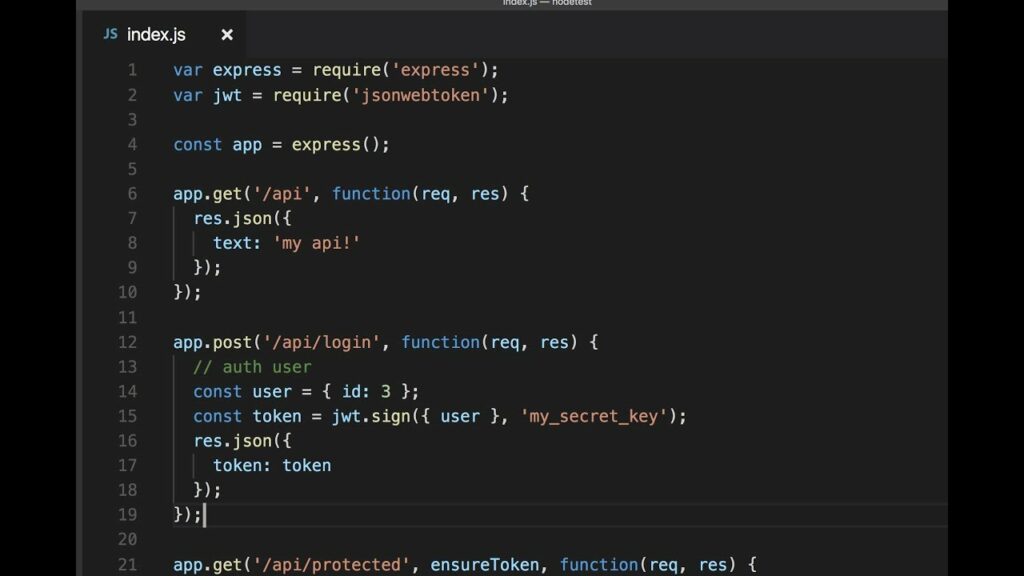
7. Implementing Authentication
For production-ready applications, adding authentication is essential for securing your API. Implementing token-based authentication using JSON Web Tokens (JWT) is a common approach. For a complete guide on adding authentication, refer to this resource on implementing authentication in your application.
“A well-designed API is both a reflection of its developer’s thought process and an enabler of seamless communication between applications.”
8. Error Handling and Validation
Effective error handling is crucial for a smooth API experience. Use middleware in Express to catch errors and return informative messages.
Example of Error Handling Middleware:
javascriptCopy codeapp.use((err, req, res, next) => {
console.error(err.message);
res.status(500).send('Internal Server Error');
});
9. Best Practices for Building APIs
- Use Proper HTTP Status Codes: Return meaningful status codes like
200 OK
,201 Created
,400 Bad Request
, and404 Not Found
. - Document Your API: Use tools like Swagger to create comprehensive API documentation.
- Ensure Security: Use HTTPS, validate user input, and implement proper authentication mechanisms.
Conclusion
Congratulations! You’ve built a basic REST API using Node.js and Express.js. This foundational setup can be expanded upon with database integration, robust authentication, and detailed error handling. REST APIs are powerful tools for enabling client-server communication, and mastering their creation is an essential skill for any full-stack developer.
If you’re ready to take your API skills further, check out more advanced topics such as implementing authentication and building a complete API with MongoDB.