Creating an API with MongoDB and Express can be a great starting point for learning full-stack development. This guide will walk you through the basics of building a simple API using these technologies. By the end, you’ll have a functional API that can handle data requests and demonstrate essential skills valuable for full-stack projects.
Why MongoDB and Express?
MongoDB and Express are powerful technologies in modern web development. MongoDB, a NoSQL database, is designed to handle large volumes of data, making it highly scalable. Express, a web application framework for Node.js, allows developers to build robust and flexible APIs efficiently.
“A well-designed API is like a universal remote – it puts you in control of the experience without knowing all the details behind it.”
By understanding these tools, full-stack developers are equipped to handle back-end tasks and create functional, dynamic applications. Discover more about essential full-stack skills here.
Setting Up Your Project
Before diving into code, let’s set up our project directory and install the necessary packages.
1. Initialize Your Project
- Open your terminal and create a new directory for your project:
bash
mkdir simple-api
cd simple-api
npm init -y - Install Express and Mongoose (a MongoDB Object Data Modeling library for Node.js):
bashCopy codenpm install express mongoose
Creating the Basic API Structure
- Create the Server File: Start by creating an
app.js
file in your project’s root folder. - Set Up Express: Add the following code to your
app.js
file to initialize Express and set up a basic server.
Javascript
const express = require(‘express’);
const mongoose = require(‘mongoose’);
const app = express();
app.use(express.json());
app.listen(3000, () => {
console.log(‘Server running on port 3000’);
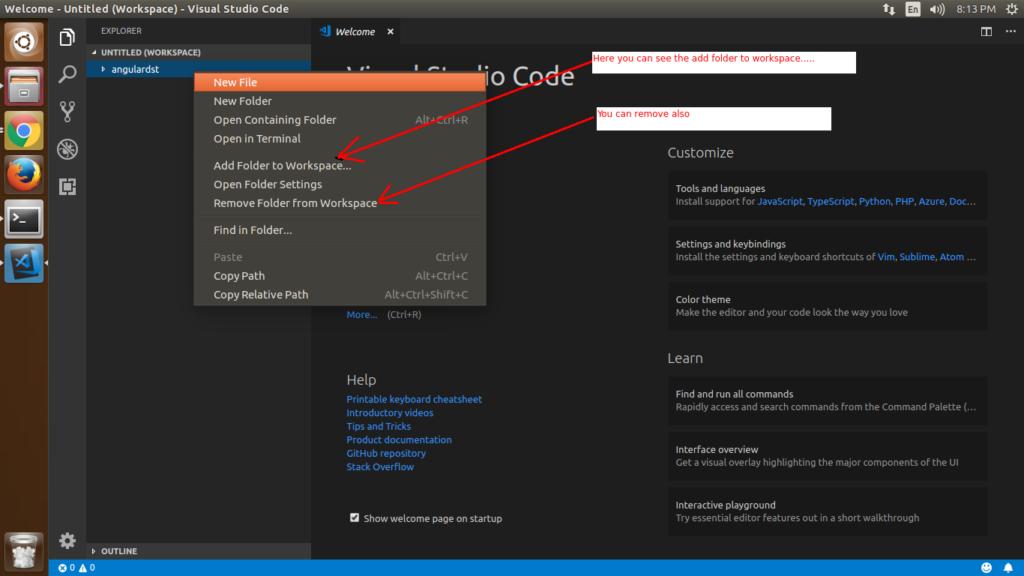
Connecting to MongoDB
To connect to MongoDB, you’ll need a MongoDB URI. MongoDB provides a free-tier option through MongoDB Atlas, which you can use to get started.
- Set Up Mongoose: Add the MongoDB connection code to your
app.js
file.javascriptCopy codemongoose.connect('your-mongodb-uri', { useNewUrlParser: true, useUnifiedTopology: true }) .then(() => console.log('MongoDB connected')) .catch((error) => console.error('Connection error:', error));
For those working in client-focused environments, it’s also essential to understand the importance of maintaining secure data management practices. Check out our guide on ensuring compliance to understand how full-stack developers can securely integrate data handling features into projects.
Defining a Model and Creating API Endpoints
With Express and MongoDB connected, the next step is to define a Mongoose model and create API endpoints. This section focuses on creating a simple CRUD (Create, Read, Update, Delete) structure for our API.
- Define the Mongoose Model: Inside a new
models
folder, create a file (e.g.,Item.js
) to define your MongoDB schema.javascriptCopy codeconst mongoose = require('mongoose'); const itemSchema = new mongoose.Schema({ name: String, description: String }); module.exports = mongoose.model('Item', itemSchema);
- Set Up the Routes: In
app.js
, add endpoints to create, read, update, and delete items.javascriptCopy codeapp.post('/items', async (req, res) => { const item = new Item(req.body); try { await item.save(); res.status(201).send(item); } catch (error) { res.status(400).send(error); } });
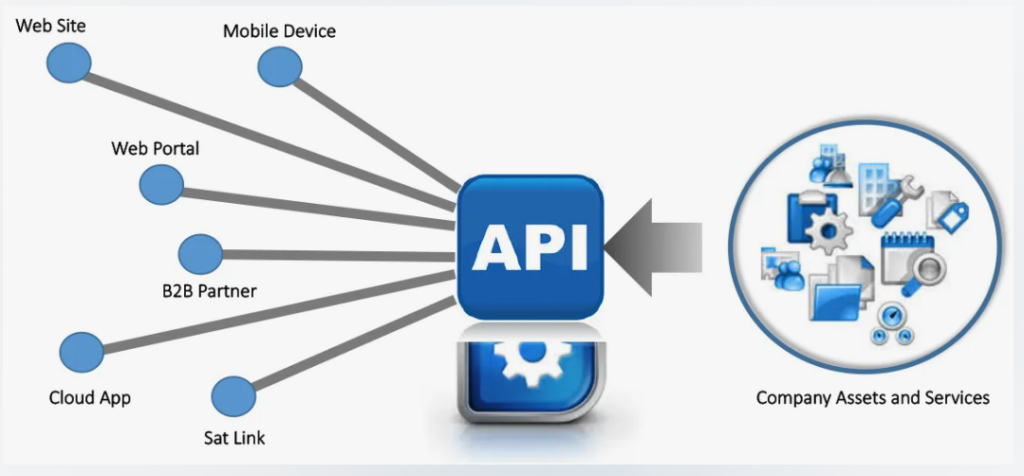
Testing and Deploying Your API
Once your API is functional, the next steps involve testing it thoroughly and considering deployment options.
When collaborating with clients or setting prices for projects involving back-end development, mastering rate negotiation is crucial. This skill can help you ensure fair compensation for the specialized work involved in building and managing APIs.
Conclusion
Building a simple API with MongoDB and Express opens the door to more advanced applications. From data handling to deployment, the skills learned here are foundational for developers looking to deepen their back-end capabilities. Remember to explore secure practices, refine your tech stack, and develop confidence in working with APIs to create reliable, high-performing applications.