JavaScript is a versatile programming language that powers dynamic behavior on websites. One of its core features is event handling — a critical component for building interactive, user-friendly web applications. Whether you are a beginner or an experienced full-stack developer, mastering event handling is essential. In this guide, we will dive into what event handling is, why it’s important, and how you can implement it effectively in your projects.
Incorporating JavaScript events allows developers to control the user experience, enhance functionality, and respond to user inputs seamlessly. To fully understand event handling, it is crucial to have a strong grasp of fundamental JavaScript concepts and a good understanding of web development basics, including HTML and CSS.
What is Event Handling in JavaScript?
Event handling refers to the process of detecting and responding to user actions such as clicks, key presses, or mouse movements on a webpage. In JavaScript, events are the signals that something has occurred, and event handlers are the functions executed in response to these events.
Example:
When a user clicks a button on a webpage, the browser generates a “click” event, and if an event handler is assigned, it will execute the corresponding JavaScript code.
An example of a simple event handler is shown below:
javascriptCopy code// Example of a click event handler
document.getElementById("myButton").addEventListener("click", function() {
alert("Button clicked!");
});
In this example, the addEventListener
method is used to listen for the click event on an element with the ID myButton
. When the user clicks the button, an alert message is displayed.
Why Event Handling is Important for Full-Stack Developmen
Event handling is crucial because it allows developers to make web applications interactive and responsive. Without event handling, webpages would be static and unable to react to user input effectively. For a comprehensive overview of skills needed for full-stack development, you can check out this guide on essential full-stack skills.
“The strength of JavaScript lies in its ability to create dynamic user experiences, and event handling is at the heart of this capability.”
— Brendan Eich, Creator of JavaScript
Types of Events in JavaScript
JavaScript events can be broadly classified into several types:
- Mouse Events: These include events like
click
,dblclick
,mouseover
,mouseout
, andmousemove
. - Keyboard Events: Such as
keydown
,keypress
, andkeyup
. - Form Events: These are events like
submit
,change
,focus
, andblur
, which occur when interacting with form elements. - Window Events: Events like
resize
,scroll
,load
, andunload
, which are related to browser window actions.
Each event type serves different purposes and can be utilized based on the user interactions your application requires.
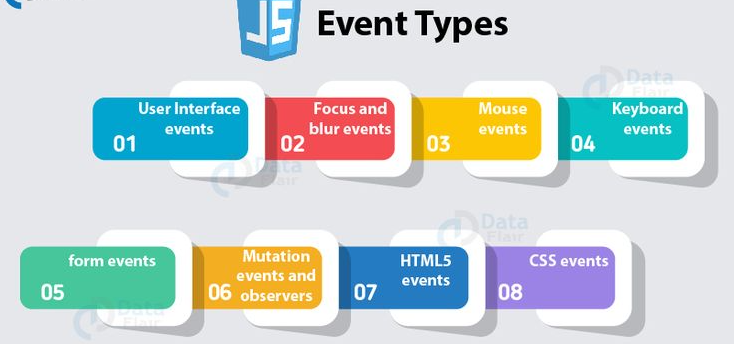
How to Use Event Listeners in JavaScript
Event listeners are methods in JavaScript that wait for an event to occur before executing a function. The addEventListener
method is the most commonly used way to handle events in modern JavaScript.
Syntax of addEventListener:
javascriptCopy codeelement.addEventListener(event, function, useCapture);
- element: The HTML element you are targeting.
- event: The event type you are listening for (e.g., “click”).
- function: The function to execute when the event occurs.
- useCapture: Optional parameter that indicates the event propagation phase.
Example:
javascriptCopy code// Using addEventListener to handle a mouseover event
const box = document.querySelector(".hover-box");
box.addEventListener("mouseover", () => {
box.style.backgroundColor = "lightblue";
});
In this example, when the user hovers over the element with the class hover-box
, its background color changes to light blue.
For more advanced JavaScript styling techniques, you can refer to this CSS3 basics guide.
Event Propagation: Capturing and Bubbling
Event propagation is the process by which events flow through the DOM. It has two main phases:
- Capturing Phase: The event starts from the top (window) and travels down to the target element.
- Bubbling Phase: The event starts from the target element and bubbles up to the top.
By default, JavaScript uses event bubbling, but you can use the useCapture
parameter in addEventListener
to control the propagation phase.
Example of Event Bubbling:
javascriptCopy code// Event bubbling example
document.querySelector(".parent").addEventListener("click", () => {
console.log("Parent element clicked");
});
document.querySelector(".child").addEventListener("click", (e) => {
console.log("Child element clicked");
e.stopPropagation(); // Prevents bubbling
});
In this example, clicking the child element stops the event from bubbling up to the parent element using e.stopPropagation()
.
“Understanding event propagation helps in building complex, responsive UI components efficiently.”
— Kyle Simpson, Author of You Don’t Know JS
Best Practices for Event Handling
- Keep Event Handlers Separate: Define your event handler functions separately rather than inline to maintain code clarity.
- Use Delegation Wisely: Event delegation helps manage events more efficiently by assigning a single event listener to a parent element rather than multiple listeners to child elements.
- Prevent Default Behavior: Use
e.preventDefault()
to prevent the browser’s default behavior for certain events, like form submissions.
Example: Prevent Default Behavior
javascriptCopy code// Preventing default form submission behavior
document.querySelector("form").addEventListener("submit", (e) => {
e.preventDefault();
console.log("Form submission prevented");
});
This code prevents the default form submission and logs a message instead.
For more insights on structuring full-stack development projects, check out this comprehensive overview of full-stack development.
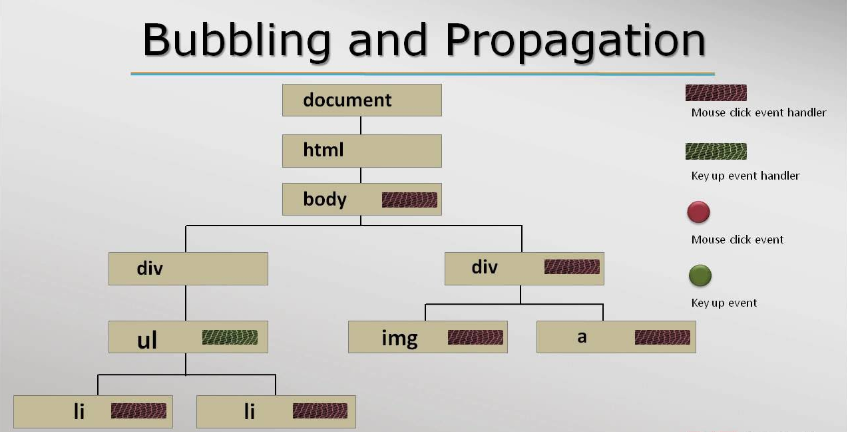
Event handling is a fundamental concept in JavaScript that allows developers to create engaging and interactive web experiences. By understanding different types of events, mastering the addEventListener
method, and applying best practices, you can significantly enhance the functionality and user experience of your web applications.
As a developer, continuously refining your event handling skills will ensure that your projects remain efficient and user-friendly. Keep exploring and experimenting with different event types and handling techniques to stay ahead in the world of full-stack development.
If you’re looking to hire skilled full-stack developers or want to expand your knowledge of essential development skills, take a look at these resources on key skills for full-stack developers.