Backend development is the foundation that powers the features and functionality of web applications. This aspect of development includes setting up and managing servers, databases, APIs, and application logic that operate behind the scenes to process data and support client-facing (frontend) applications. Whether you’re looking to develop a simple website or a complex web application, understanding backend development is essential for creating efficient and scalable solutions. This guide offers an overview of key components, including server setup, database integration, and core backend technologies.
“Backend development forms the backbone of any web application, enabling secure, efficient, and scalable data processing.”
Key Technologies in Backend Development
Backend development involves several technologies, each with a specific role in handling the “behind-the-scenes” functions of an application. Here are some of the primary elements in a backend developer’s toolkit:
- Servers and Hosting: The server is the environment where applications run. Servers host code, databases, and APIs, and can be set up on local machines or on cloud platforms like AWS, Google Cloud, or Azure.
- Programming Languages: Popular languages for backend development include JavaScript (Node.js), Python, Java, Ruby, and PHP. Each language has frameworks and libraries that simplify backend processes, such as Express for Node.js, Django for Python, and Ruby on Rails.
- Databases: Databases store and manage application data. Backend developers often use SQL databases like MySQL and PostgreSQL for relational data structures, and NoSQL databases like MongoDB for flexible, document-based storage. For an introduction to database management, check out this overview of SQL vs. NoSQL databases.
- APIs (Application Programming Interfaces): APIs enable communication between different parts of an application and with external services. RESTful APIs and GraphQL are common protocols used to structure and request data from the backend.
Server-Side Scripting and APIs
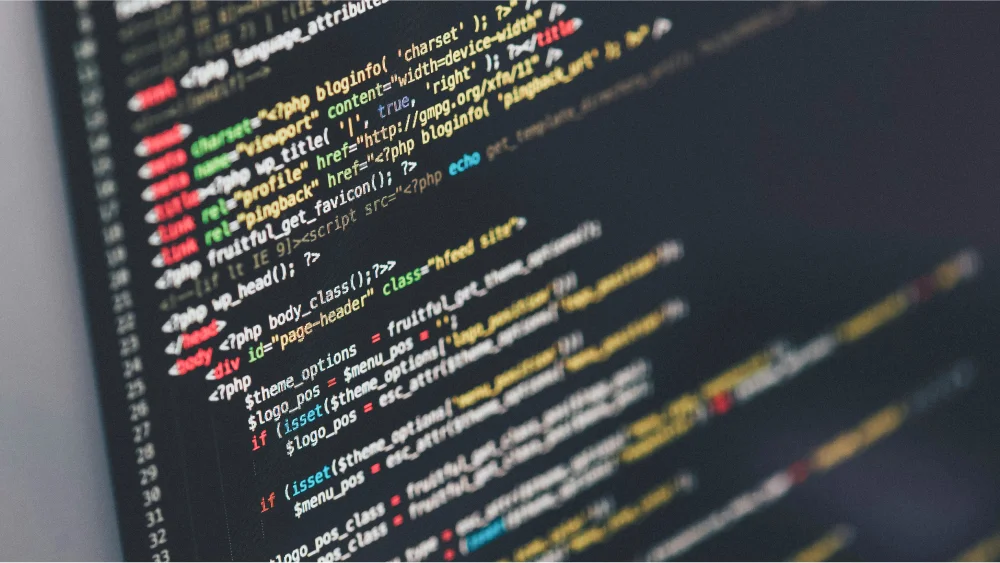
One of the primary roles of backend development is server-side scripting. This is the code that handles business logic, processes data, and responds to client requests. Server-side scripting can be used to manage user accounts, process payments, or interact with databases. Node.js, a powerful runtime environment for JavaScript, is widely used for creating server-side scripts in modern web applications.
APIs (Application Programming Interfaces) play a crucial role in backend development. APIs allow different software components to communicate, letting the frontend interact seamlessly with backend resources. RESTful APIs are the most common type, designed to work over HTTP and provide structured access to data. In Node.js, the Express framework is frequently used to set up and manage these APIs.
Database Integration
Databases are essential for storing and managing data. Depending on the application’s requirements, you may use a relational (SQL) or a non-relational (NoSQL) database.
- Relational Databases (SQL): SQL databases like MySQL and PostgreSQL use tables to store data with structured relationships. They are ideal for applications that require a strong schema, such as financial systems or e-commerce stores.
- Non-relational Databases (NoSQL): NoSQL databases like MongoDB store data in a more flexible format, often as JSON-like documents. This flexibility is particularly advantageous in applications where data structure may evolve over time, such as social media platforms.
If you’re working with MongoDB, check out this guide on CRUD operations in MongoDB with Node.js.
Backend Frameworks and Libraries
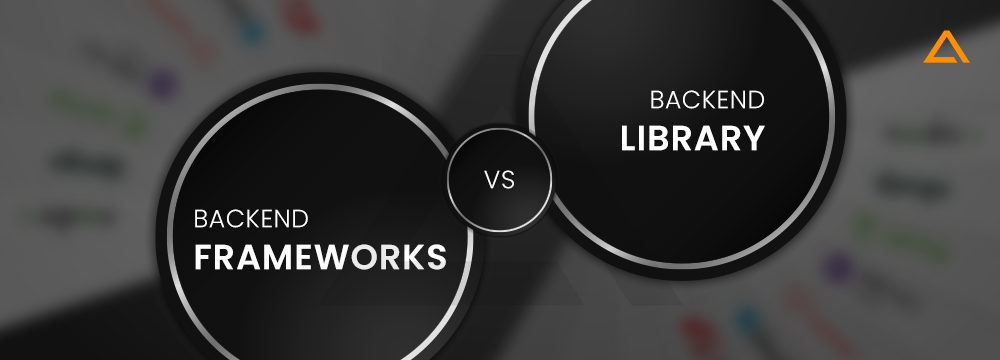
Frameworks and libraries streamline backend development by providing pre-built code modules that support essential tasks like routing, database connections, and data handling. Some popular backend frameworks include:
- Express.js: A minimalist framework for Node.js, commonly used for building APIs and web applications.
- Django: A high-level Python framework that promotes rapid development and clean, pragmatic design.
- Ruby on Rails: Known for its simplicity and speed, Rails is popular in the startup world.
Each framework has unique features and strengths, making it suitable for various use cases.
Backend Security and Best Practices
Security is critical in backend development, particularly for applications handling sensitive data, such as personal information or payment details. Backend security measures include:
- Authentication and Authorization: Verifying users’ identities and managing their access rights.
- Data Encryption: Encrypting data both in transit and at rest to protect it from unauthorized access.
- Input Validation: Ensuring only valid data reaches the server to prevent attacks like SQL injection.
By adopting these practices, backend developers help protect both the application and its users.
Conclusion
Backend development is foundational to creating functional, secure, and scalable applications. From server management and database integration to setting up APIs and ensuring security, backend developers build the structures that enable seamless user experiences. For those new to backend development, mastering JavaScript’s modern features is an excellent start. Here’s a resource on key JavaScript features in ES6 that can enhance your coding efficiency as you work with backend frameworks like Node.js.