Express.js is a fast, minimal, and flexible Node.js web application framework that simplifies building robust APIs. Whether you’re working on a simple backend for a mobile app or a complex, multi-service architecture, Express.js provides a powerful toolkit to manage server-side tasks seamlessly. This guide will introduce you to Express.js and outline how to use it effectively for API development.
What is Express.js?
Express.js is a web application framework for Node.js, known for its simplicity and speed. It helps developers manage HTTP requests, routing, and middleware in a straightforward way. Express.js is perfect for both small and large applications, and it provides the flexibility to build any kind of web service or RESTful API.
“Express.js allows developers to concentrate on building business logic rather than worrying about the complexities of network communication.”
This level of abstraction makes Express.js particularly popular among developers seeking an efficient way to build APIs.
Setting Up an Express.js API
To get started with Express.js, first install Node.js and then initialize a new Node project. You can install Express.js through the Node package manager (npm) with the following command:
bashCopy codenpm install express
After installation, create an initial server setup. Here’s a basic example of an Express server:
javascriptCopy codeconst express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, Express!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code initializes a basic Express server that listens on port 3000 and sends a response when accessing the root route.
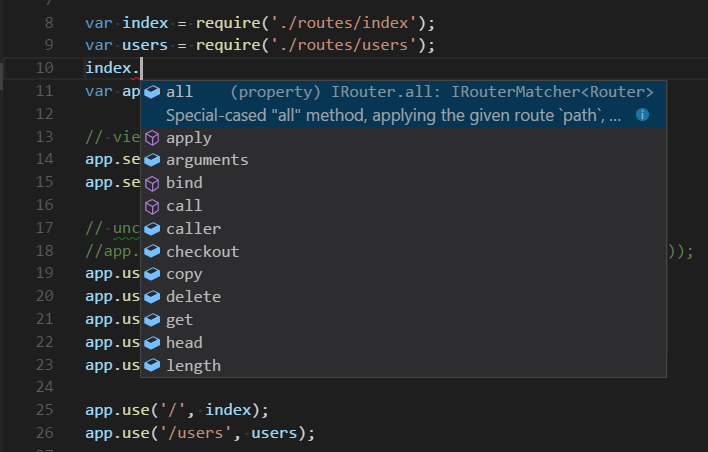
Organizing Routes and Controllers
One of the primary benefits of Express.js is its modularity. In a real-world application, it’s best to separate routing and controller logic for scalability. This allows for cleaner code organization and better debugging.
For example, you can create a route file for user-related routes and another for product-related routes. By modularizing your routes, your code remains clean and manageable even as your application grows.
Optimizing Express.js APIs for Performance
Building an efficient API is crucial to maintaining performance and ensuring a smooth user experience. Techniques such as caching and proper database indexing are essential for optimizing Express applications. For a deeper dive into performance optimization, check out Optimizing Full Stack Applications for Performance, which covers advanced techniques for maximizing API efficiency.
Caching Strategies for Better Performance
Caching helps to reduce server load and latency, improving the overall response time of your API. Implementing caching strategies can drastically enhance the performance of your Express.js application, especially when handling high volumes of traffic. To understand caching methods in more detail, you can explore Caching Strategies in Full Stack Development, which offers practical insights into leveraging cache layers to boost application performance.
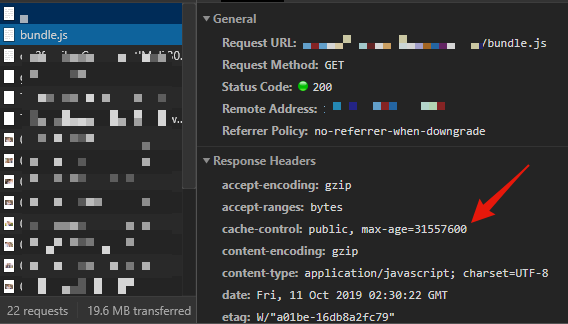
Integrating Git for Collaborative Development
When building APIs with Express.js, collaboration often involves using Git. Mastering Git commands and workflows, such as branching and rebasing, can enhance teamwork and productivity on large projects. For a comprehensive guide on collaborative Git practices, including how to use branching, rebasing, and cherry-picking to manage code efficiently, check out Mastering Git Collaboration.
Conclusion
Express.js is a powerful framework for building APIs efficiently. By understanding the basics of setup, modular routing, performance optimization, and caching, you can harness the full potential of Express.js. Remember, maintaining a smooth development process involves not only writing efficient code but also implementing performance optimizations and collaborative workflows.
In summary, Express.js provides developers with the tools necessary for creating scalable, high-performance APIs, making it an invaluable addition to any developer’s toolkit.