In modern JavaScript development, testing plays a crucial role in ensuring code quality and reliability. Whether you’re building a web application or an API, understanding testing frameworks like Jest and Mocha can significantly improve your development process. Let’s explore how to get started with testing in JavaScript and why it’s an essential skill for developers.
Why Testing Is Important for JavaScript Development
Testing your JavaScript code is vital because it helps catch bugs early, ensures code functionality, and builds confidence in your software. In the context of full-stack development, having strong testing skills is a must. When posting job requirements for full-stack developers, employers often look for candidates who are proficient in testing frameworks. To learn more about essential skills for full-stack developers, check out this comprehensive guide.
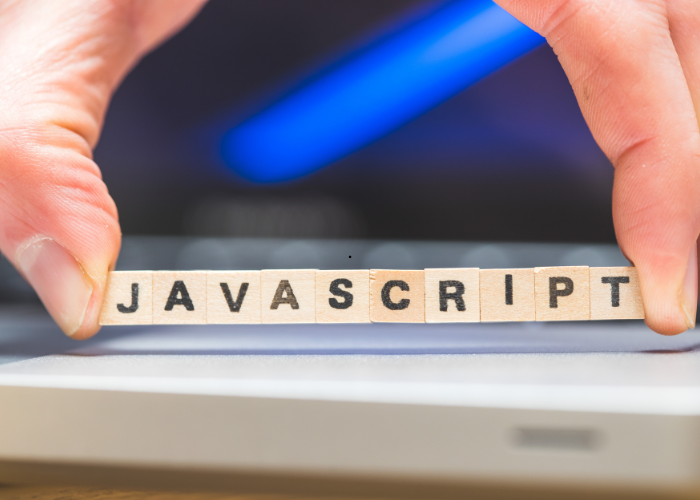
An Overview of Jest
Jest is a popular testing framework created by Facebook. It is widely used for testing JavaScript applications, particularly those built with React. Jest is known for its simplicity, fast performance, and powerful features like snapshot testing, which can detect unexpected changes in the UI.
“Testing is not just a ‘nice-to-have’ — it’s an integral part of building scalable and maintainable software solutions.” — Senior Full-Stack Developer
Key Features of Jest
- Zero configuration: Easy setup and minimal configuration.
- Snapshot testing: Useful for testing UI components.
- Mocking capabilities: Allows you to simulate functions and modules.
When to Use Mocha
While Jest is great for React applications, Mocha is often preferred for projects that require more flexibility. Mocha is a feature-rich JavaScript testing framework that runs on Node.js. It’s commonly used with libraries like Chai for assertions, providing a more customizable testing experience.
Mocha is also well-suited for testing APIs, which is a critical task for full-stack developers who handle both client-side and server-side development. If you’re looking to expand your knowledge of key technologies used by full-stack developers, visit this detailed article.
Setting Up Your Testing Environment
To get started with testing in JavaScript, follow these steps:
- Install Jest:bashCopy code
npm install --save-dev jest
- Install Mocha:bashCopy code
npm install --save-dev mocha
Image Suggestion 1:
Add an image here showing the terminal commands for installing Jest and Mocha. This visual representation will help beginners understand the setup process.
Writing Your First Test with Jest
Let’s write a simple test case using Jest:
javascriptCopy code// sum.js
function sum(a, b) {
return a + b;
}
module.exports = sum;
javascriptCopy code// sum.test.js
const sum = require('./sum');
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
Running the test with npx jest
should give you a passing result if everything is set up correctly.
Image Suggestion 2:
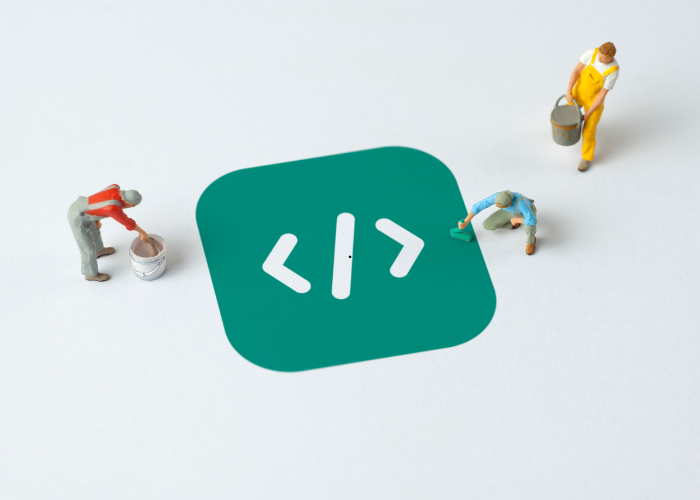
Using Mocha for API Testing
Mocha’s flexibility makes it a great choice for testing APIs. You can combine it with Chai for better assertions. For example:
javascriptCopy codeconst chai = require('chai');
const expect = chai.expect;
describe('API Test', function () {
it('should return status 200', function (done) {
// Example API request using a hypothetical function
apiRequest('/endpoint', (response) => {
expect(response.status).to.equal(200);
done();
});
});
});
For full-stack developers, managing APIs effectively is crucial. Understanding how to write efficient API tests is just as important as learning the best practices for handling databases and APIs.
Conclusion
Testing in JavaScript using frameworks like Jest and Mocha not only improves code quality but also saves time by catching issues early. As a developer, mastering these tools will make you more efficient and better prepared for building robust applications.
If you’re interested in diving deeper into the essential technologies for full-stack development or improving your skills in handling APIs, check out the linked resources for more insights.