JavaScript DOM (Document Object Model) manipulation is a crucial skill for any front-end developer. It allows developers to interact with and modify the structure, style, and content of web pages dynamically. Whether you’re creating a new element, updating text, or responding to user interactions, understanding DOM manipulation is essential for building interactive and user-friendly websites.
What is DOM Manipulation?
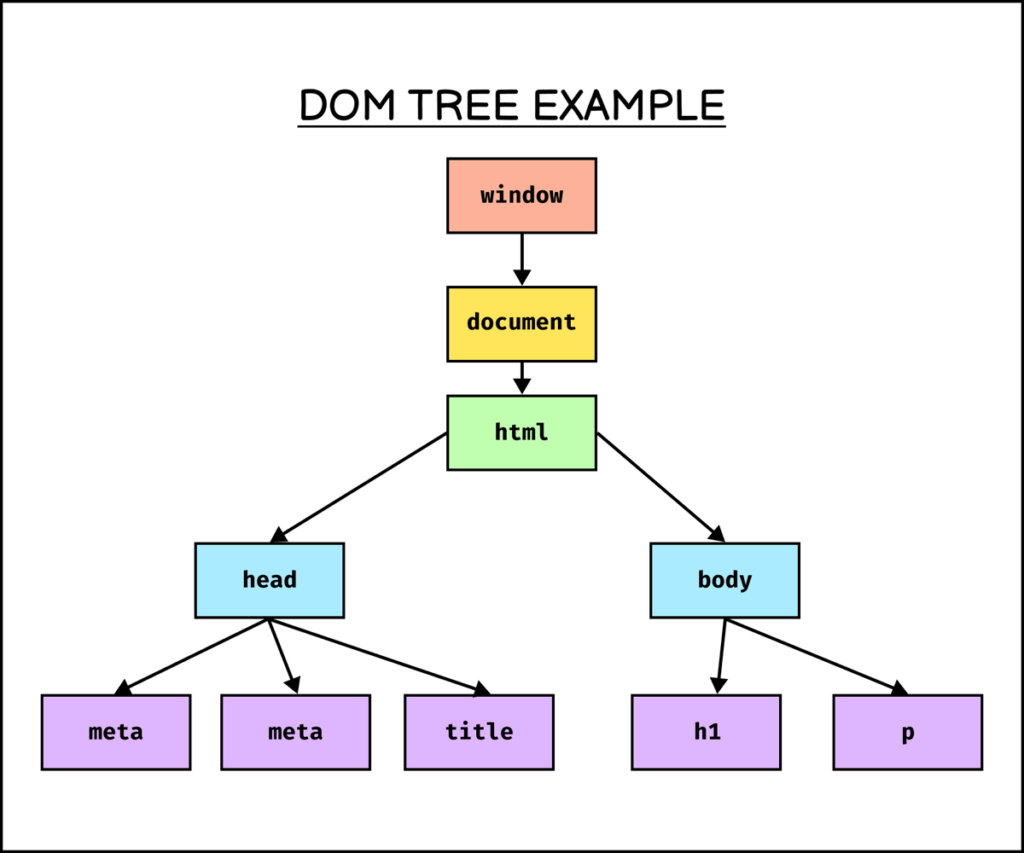
The DOM represents the hierarchical structure of a webpage as a tree of objects. JavaScript allows developers to access and manipulate these objects to create dynamic user experiences. Some common DOM manipulation tasks include:
- Adding, removing, or updating elements.
- Changing styles dynamically.
- Handling user events like clicks and keypresses.
- Managing animations and transitions.
To make the most out of these techniques, it’s essential to familiarize yourself with common front-end developer tools that simplify debugging and optimize your development process.
Key Techniques in DOM Manipulation
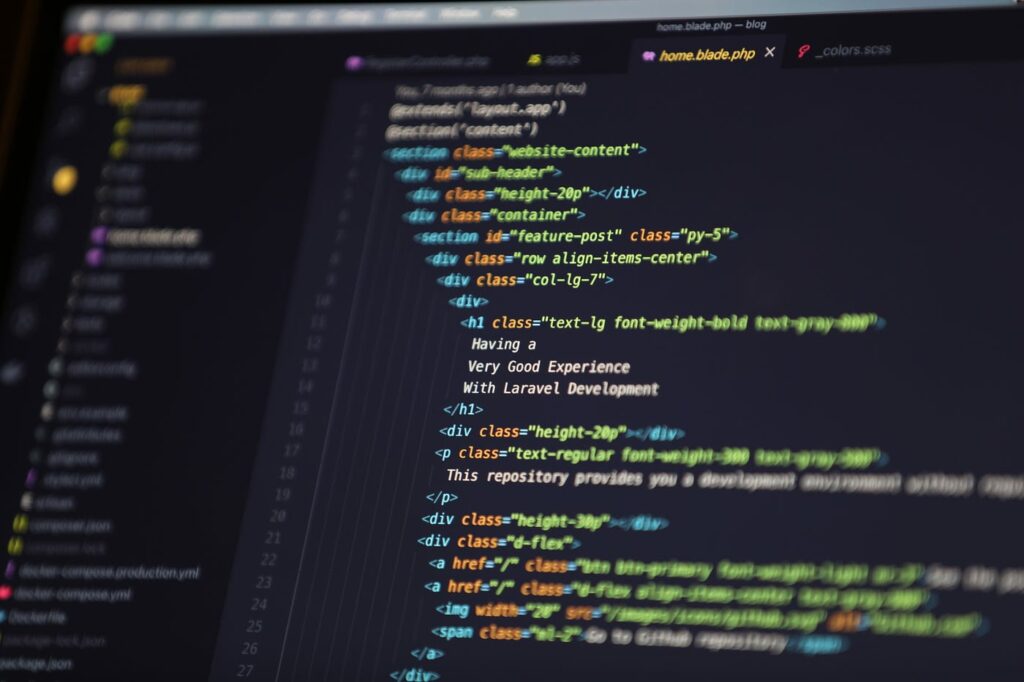
- Selecting Elements
Use methods likegetElementById
,querySelector
, andgetElementsByClassName
to select DOM elements. For example:const button = document.querySelector('#myButton');
- Creating New Elements
Dynamically add elements to your webpage usingcreateElement
andappendChild
. For instance:const newDiv = document.createElement('div'); newDiv.textContent = 'Hello, DOM!'; document.body.appendChild(newDiv);
- Event Handling
Attach event listeners to elements to respond to user actions.button.addEventListener('click', () => { alert('Button clicked!'); });
- Style Manipulation
Change element styles dynamically using thestyle
property.newDiv.style.color = 'blue'; newDiv.style.fontSize = '20px';
“Good developers know how to write code. Great developers know how to simplify complex processes.” – This wisdom applies perfectly to mastering DOM manipulation, as it’s all about streamlining the interaction between code and the user interface.
For better project management and visibility, consider checking out the best time to post a job on Efrelance for maximum reach to ensure you find skilled front-end developers who excel in DOM manipulation.
Benefits of Mastering DOM Manipulation
- Enhances interactivity and user experience.
- Enables real-time updates to the webpage.
- Powers dynamic features like modals, sliders, and form validations.
Advanced Techniques
As your projects grow, you’ll encounter scenarios requiring advanced techniques like virtual DOM usage in frameworks such as React. Understanding the basics of JavaScript DOM manipulation makes transitioning to such frameworks seamless. If you aim to build SEO-friendly websites, these essential SEO tips for front-end developers can help enhance your DOM practices.
Conclusion
JavaScript DOM manipulation is the foundation of interactive web development. By mastering these skills, you can create dynamic, responsive, and engaging web applications. Remember, the journey doesn’t end here; keep exploring and applying these concepts to build robust projects.