JavaScript plays a vital role in modern web development, especially when it comes to form validation. It ensures that user inputs meet specific criteria before being sent to the server, enhancing both the user experience and application security. In this post, we’ll explore how JavaScript handles form validation, tips for effective implementation, and essential tools for building robust forms.
Why Form Validation is Crucial
Form validation ensures the data users enter is clean, accurate, and secure. Without validation, applications risk processing incomplete or malicious inputs, leading to potential errors and security vulnerabilities. JavaScript provides the flexibility to validate forms in real time, giving users instant feedback and reducing server load.
Incorporating tools like JavaScript libraries such as jQuery can streamline the validation process. Learn more about these libraries in Introduction to JavaScript Libraries: jQuery, which outlines their features and how they simplify coding.
Types of JavaScript Form Validation
Client-Side Validation happens in the user’s browser before the form is submitted. It’s faster and provides immediate feedback, but should be complemented with server-side validation for security.
Server-Side Validation ensures that even if malicious users bypass client-side validation, your backend still verifies the data.
For beginners in web development, understanding how to integrate JavaScript with modern tools is vital. Check out Introduction to Web APIs to explore how APIs can enhance your form validation processes.
Common Validation Methods
- Required Fields: Ensure specific fields are not left blank.
- Input Length: Check the minimum or maximum number of characters.
- Regular Expressions: Validate formats like email addresses, phone numbers, or zip codes.
- Error Messages: Provide clear instructions when inputs don’t meet the criteria.
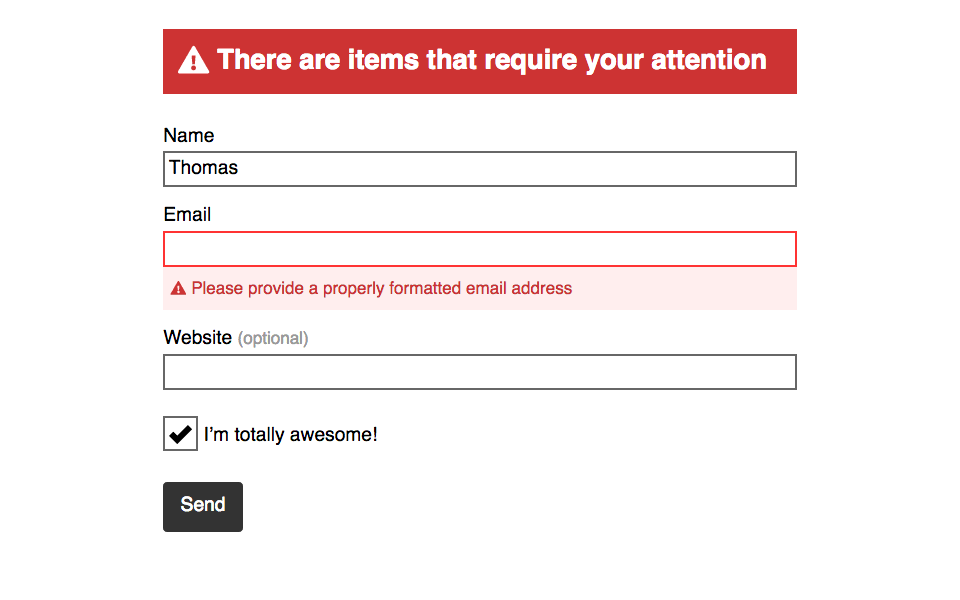
“Clear error messages make validation user-friendly, turning mistakes into learning moments.”
Step-by-Step Guide to JavaScript Form Validation
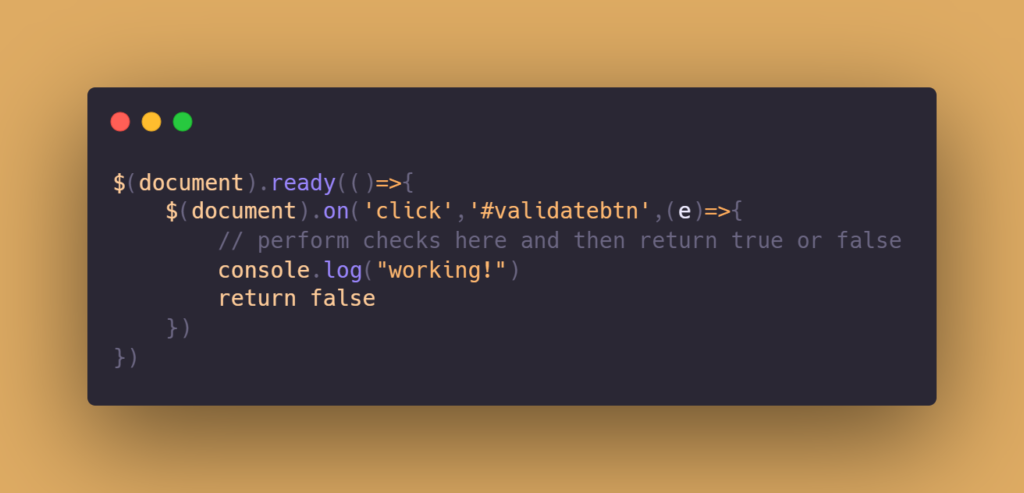
Here’s an example of validating a simple email form:
javascriptCopy codeconst form = document.getElementById("myForm");
const email = document.getElementById("email");
const error = document.getElementById("error");
form.addEventListener("submit", (e) => {
if (!validateEmail(email.value)) {
error.textContent = "Please enter a valid email address.";
e.preventDefault();
}
});
function validateEmail(email) {
const regex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
return regex.test(email);
}
}
Testing Your Validation
Testing is crucial to ensure your validation logic is robust. For insights into efficient testing practices, visit A Beginner’s Guide to Testing in Front-End Development. It explains various methods and tools to debug and enhance your code.
Conclusion
JavaScript form validation is a cornerstone of user-friendly and secure web applications. By combining client-side and server-side validation, using modern libraries, and rigorously testing your forms, you can build seamless experiences for users. Start implementing these practices today and see the difference they make in your projects!