JavaScript functions are the core building blocks of any modern web application. They allow you to encapsulate code into reusable units, making your programs cleaner, more modular, and easier to manage. If you’re new to JavaScript, understanding how functions work is one of the most important skills to develop.
As a developer once said:
“Functions are like recipes for a chef—follow the steps, and you’ll get consistent results every time.”
What is a JavaScript Function?
A function is a reusable block of code that performs a specific task. You define a function once and can call it as many times as needed.
Here’s a basic syntax of a function in JavaScript:
javascriptCopy codefunction functionName(parameters) {
// Code to execute
}
Why Use Functions?
- Reusability: Write once, use anywhere.
- Modularity: Break complex tasks into smaller, manageable pieces.
- Maintainability: Easier to debug and maintain code.
Explore more foundational topics in web development with Mastering CSS Basics: The Key to Stunning Web Design.
Types of Functions in JavaScript
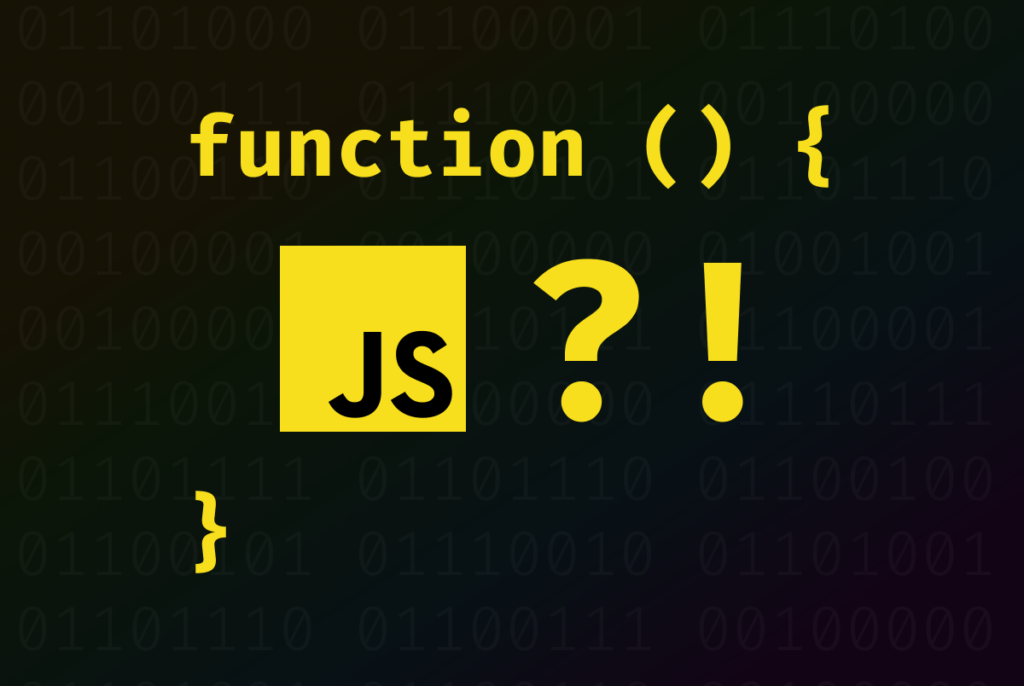
- Function Declarations
These are the most common and traditional way to define a function.javascriptCopy codefunction greet(name) { return `Hello, ${name}!`; } console.log(greet("Alice")); // Output: Hello, Alice!
- Function Expressions
Functions can also be assigned to variables.javascriptCopy codeconst add = function(a, b) { return a + b; }; console.log(add(2, 3)); // Output: 5
- Arrow Functions
A concise way to write functions introduced in ES6.javascriptCopy codeconst multiply = (x, y) => x * y; console.log(multiply(4, 5)); // Output: 20
- Anonymous Functions
Functions without names, often used as arguments.javascriptCopy codesetTimeout(function() { console.log("Hello, World!"); }, 1000);
- Immediately Invoked Function Expressions (IIFE)
These functions execute immediately after being defined.javascriptCopy code(function() { console.log("This runs immediately!"); })();
Learn more about enhancing JavaScript performance with Website Performance Optimization and other tools for efficient coding.
Parameters and Arguments
Functions can accept inputs, known as parameters, and use them to customize outputs.
javascriptCopy codefunction calculateArea(length, width) {
return length * width;
}
console.log(calculateArea(5, 10)); // Output: 50
Scope and Closures
- Scope
Variables inside a function are local to that function.javascriptCopy codefunction showScope() { let localVar = "I'm local"; console.log(localVar); } showScope(); // console.log(localVar); // Error: localVar is not defined
- Closures
Functions can “remember” the variables from their outer scope.javascriptCopy codefunction outer() { let outerVar = "Outer"; return function inner() { console.log(outerVar); }; } const innerFunc = outer(); innerFunc(); // Output: Outer
Function Best Practices
- Keep Functions Small: Focus on one task per function.
- Use Descriptive Names: Make function names self-explanatory.
- Avoid Global Variables: Keep variables local to reduce conflicts.
- Use Default Parameters: Provide default values for parameters when applicable.javascriptCopy code
function greet(name = "Guest") { return `Hello, ${name}!`; } console.log(greet()); // Output: Hello, Guest!
Expand your knowledge by learning about HTML Semantic Elements and JavaScript Events for dynamic web interactions.
Real-World Example
Consider an e-commerce site where users calculate the total price of items in their cart
javascriptCopy codefunction calculateTotal(items) {
return items.reduce((total, item) => total + item.price, 0);
}
const cart = [
{ name: "Shirt", price: 20 },
{ name: "Pants", price: 40 },
{ name: "Hat", price: 10 }
];
console.log(`Total: $${calculateTotal(cart)}`); // Output: Total: $70
With functions, you can create modular and reusable code for tasks like form validation, data processing, or event handling.
Master the art of writing efficient functions, and you’ll unlock the potential to build scalable and maintainable JavaScript applications!