In React development, managing state and props efficiently is key to building responsive and dynamic applications. By understanding how state and props work together, developers can create seamless, interactive user experiences. This guide dives into effective practices for state and props management, with tips for ensuring data flows smoothly throughout your application.
“A well-managed state is the backbone of a stable and responsive application.”
Understanding State and Its Role
State in React represents the data or “state” of a component at a given time. It can change dynamically based on user interactions or API responses, updating the UI automatically. For instance, in a shopping cart app, the items and total cost displayed in the cart would be managed as state variables.
Using useState
and useReducer
for managing state enables developers to fine-tune application behavior. Additionally, these hooks help manage complex state changes, especially in applications with multiple interdependent components. For more on managing events that can affect state, check out this comprehensive guide to mastering JavaScript events.
Props: Passing Data Across Components
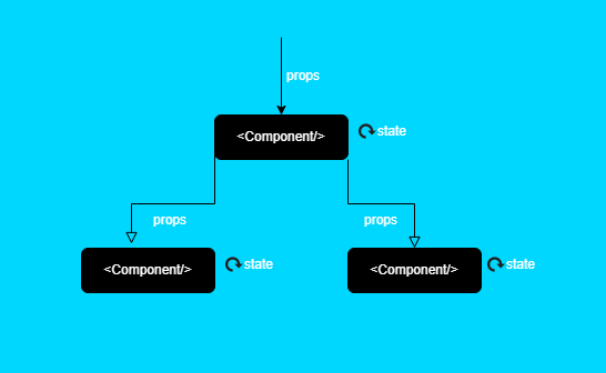
Props, short for “properties,” allow data to be passed from a parent component to its children. Unlike state, props are immutable, meaning a child component cannot directly modify them. Props are ideal for passing configuration data or callback functions to components, keeping the application modular and organized.
In larger applications, lifting state to a common parent component and using props to share it with child components can help avoid redundant state across components. This approach keeps data centralized and minimizes unexpected behaviors in the UI.
Practical Tips for Effective State and Props Management
- Keep State Local Whenever Possible: If only one component uses a piece of state, avoid lifting it to a parent component unnecessarily. This keeps your code cleaner and enhances component reusability.
- Use Derived State Carefully: Avoid duplicating data in state that can be derived from existing state or props. This reduces errors and makes debugging easier.
- Avoid Prop Drilling: If multiple levels of components need access to a particular piece of state, consider using context or global state management libraries like Redux. This keeps your code from becoming tangled and difficult to maintain.
For a deeper look at managing complex state changes, the eFrelance article What is a Full Stack Developer: Skills and Expertise offers insights into how full-stack developers handle data flow in advanced applications.
Common Pitfalls to Avoid
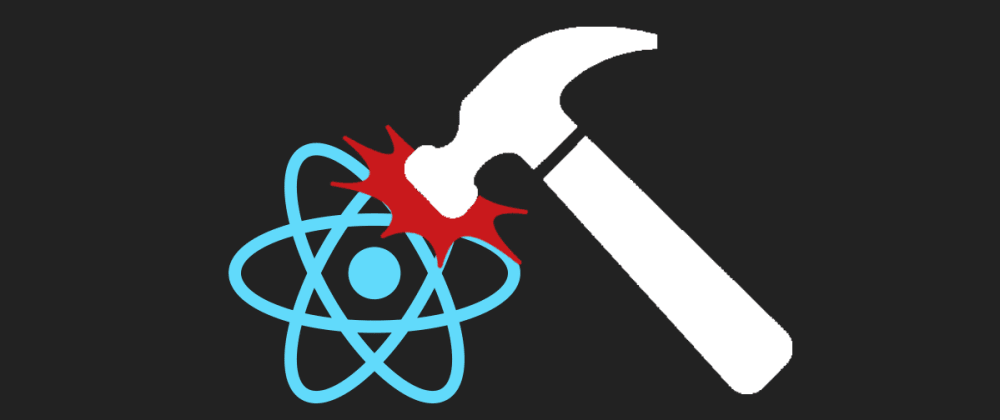
Managing state and props efficiently also means being aware of potential pitfalls. Some common issues include:
- Overusing Global State: Not every piece of data needs to be accessible across the entire application. Determine whether each state variable is best kept local or lifted.
- Inconsistent Data Flows: Ensure data dependencies are clearly defined, especially when a component relies on multiple sources of props or state.
- Re-Renders: Overusing state can lead to unnecessary re-renders, affecting performance. Keep your components lean by optimizing which states trigger updates.
Conclusion
Mastering state and props management in React is crucial for building dynamic applications that provide a smooth user experience. By keeping state local where possible, using props effectively, and avoiding common pitfalls, developers can ensure data flows logically and efficiently throughout their application.