Unit testing is a fundamental practice in software development, especially for full-stack developers working with React on the frontend and Node.js on the backend. Unit tests ensure that individual components or functions work as expected, helping to prevent bugs and maintain code quality. In this post, we’ll explore why unit testing is essential, the tools used, and how to implement it effectively in both React and Node.js projects.
What is Unit Testing?
Unit testing involves testing small, isolated parts of your application. Each unit test validates a specific function or component independently, ensuring it behaves correctly under various scenarios. This method offers numerous benefits:
- Detects bugs early in the development cycle
- Ensures code reliability and maintainability
- Facilitates refactoring with confidence
For full-stack developers, mastering unit testing is crucial. Understanding its importance can enhance your job readiness, as highlighted in the article “Important Skills to Include in Your Job Post for Full-Stack Developers”.
Unit Testing Tools for React and Node.js
To effectively perform unit testing in React and Node.js, you need the right set of tools:
- Jest: A popular testing framework for JavaScript applications. It is often used for both React and Node.js projects because of its simplicity and comprehensive feature set.
- Mocha: A flexible testing library for Node.js, known for its ease of integration with various assertion libraries like Chai.
- React Testing Library: Designed specifically for testing React components, it focuses on testing user interactions rather than implementation details.
“A good unit test should be deterministic and isolated, providing a reliable safety net for your codebase.” — Anonymous Developer
How to Write Unit Tests in React
React applications are built using components, making them easier to test in isolation. Here’s a basic example of a unit test using Jest and React Testing Library.
jsxCopy codeimport { render, screen } from '@testing-library/react';
import MyComponent from './MyComponent';
test('renders the component with the correct text', () => {
render(<MyComponent />);
const element = screen.getByText(/Hello, World!/i);
expect(element).toBeInTheDocument();
});
In this example, the test checks whether the component renders the text “Hello, World!” correctly.
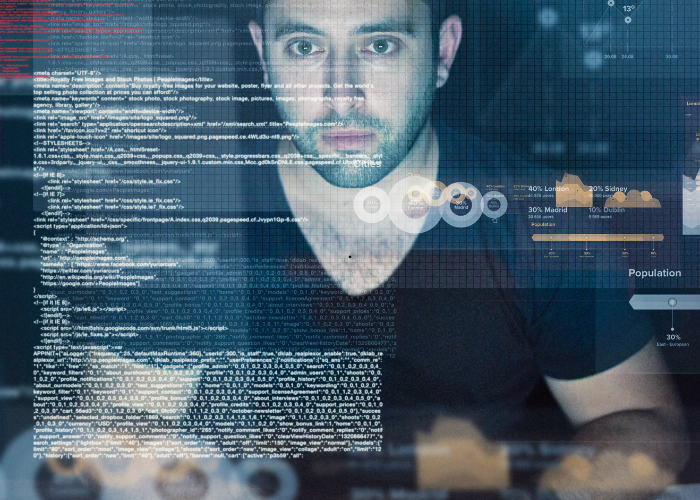
Image Idea: A diagram showing the structure of a React component test, highlighting the process of rendering, querying, and asserting.
Placement: Place this image right after the React unit testing code snippet to provide a visual aid for understanding the testing process.
Writing Unit Tests for Node.js
Node.js applications often involve testing functions, middleware, or API routes. Here’s an example of a basic unit test using Mocha:
javascriptCopy codeconst assert = require('assert');
const addNumbers = (a, b) => a + b;
describe('Addition Function', () => {
it('should return the sum of two numbers', () => {
assert.strictEqual(addNumbers(2, 3), 5);
});
});
This test ensures that the addNumbers
function correctly returns the sum of two numbers.
When working with Node.js and managing databases, knowing how to integrate unit tests effectively is vital. Learn more about the key technologies full-stack developers should know here.
Best Practices for Unit Testing
- Keep Tests Isolated: Each test should focus on one unit of functionality and should not depend on other tests.
- Mock External Dependencies: Use mocks and stubs to simulate API calls or database queries. This approach ensures tests run quickly and do not rely on external factors.
- Write Readable Tests: Tests should be easy to read and understand. A well-written test serves as documentation for your code.
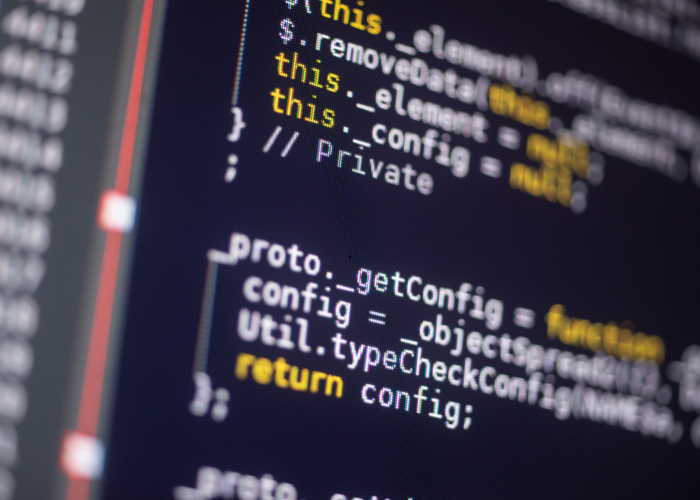
Image Idea: A flowchart illustrating the process of unit testing in a Node.js application, from setting up tests to mocking dependencies.
Placement: Add this image after the “Best Practices for Unit Testing” section to give a visual representation of the testing workflow.
Integrating Unit Testing into Your Full-Stack Workflow
Incorporating unit tests into your development workflow streamlines the debugging process and makes the integration of frontend and backend components smoother. By utilizing tools like Jest for React and Mocha for Node.js, you can confidently refactor your code and ensure consistent functionality across your stack.
For a deeper understanding of how full-stack developers efficiently manage databases and APIs, explore this insightful article on managing databases and APIs efficiently.
Conclusion
Unit testing is a powerful technique that every full-stack developer should master. It not only boosts the quality of your React and Node.js applications but also provides a solid foundation for building reliable and scalable software solutions. Start incorporating unit tests into your projects today to experience the benefits firsthand!