In today’s dynamic web development landscape, managing data efficiently is crucial for building robust applications. MongoDB, paired with Node.js, offers a powerful combination for handling data with a flexible, non-relational approach. Whether you’re developing a small project or a large-scale application, understanding how to perform CRUD (Create, Read, Update, Delete) operations with MongoDB in Node.js is essential.
In this guide, we’ll walk you through everything you need to get started with MongoDB and Node.js, covering how to set up your environment, connect to MongoDB, and implement each CRUD operation step by step.
1. Setting Up Your Environment
Before diving into CRUD operations, let’s set up our environment.
Prerequisites:
- Node.js installed on your system. If you’re new to Node.js, refer to this introduction to Node.js and its uses to understand its basics and why it’s a popular choice for backend development.
- MongoDB installed locally or access to MongoDB Atlas (cloud-based MongoDB).
- Basic knowledge of JavaScript and command line operations.
Installing Required Packages
To start, initialize your Node.js project by running:
bashCopy codenpm init -y
Next, install the necessary packages:
bashCopy codenpm install express mongodb
- Express: A lightweight framework for Node.js that simplifies backend development.
- MongoDB: The official Node.js driver for connecting and interacting with MongoDB databases.
2. Connecting Node.js with MongoDB
To interact with MongoDB, you need to establish a connection from your Node.js application. Let’s create a simple index.js
file.
javascriptCopy codeconst express = require('express');
const { MongoClient } = require('mongodb');
const app = express();
const PORT = 3000;
const uri = 'mongodb://127.0.0.1:27017';
const client = new MongoClient(uri);
app.use(express.json());
client.connect()
.then(() => {
console.log('Connected to MongoDB');
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
})
.catch((error) => console.error('Connection error:', error));
This code initializes an Express server and connects to a MongoDB database. If you’re setting up your first server, this guide on setting up your first Node.js server provides a helpful overview.
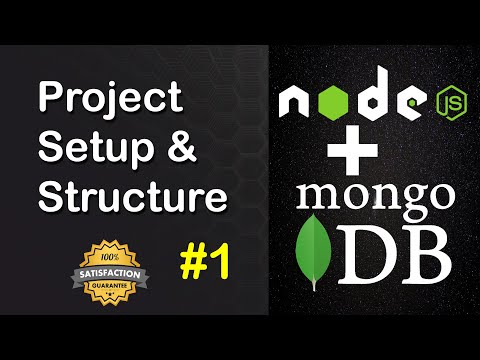
3. CRUD Operations Explained
Now, let’s dive into the heart of the article: performing CRUD operations with MongoDB in a Node.js application.
3.1. Create Operation (Insert Data)
The Create operation allows you to add new documents to your MongoDB collection. Let’s implement a POST endpoint to create a new record.
javascriptCopy codeapp.post('/api/users', async (req, res) => {
const user = req.body;
try {
const db = client.db('mydatabase');
const result = await db.collection('users').insertOne(user);
res.status(201).send(result);
} catch (error) {
res.status(500).send({ error: 'Failed to create user' });
}
});
This endpoint receives user data from the request body and inserts it into the users
collection. If you’re building a simple API and want more insight into working with MongoDB and Express, explore this step-by-step guide on building a simple API.
3.2. Read Operation (Fetch Data)
The Read operation retrieves data from the database. Here’s how to create a GET endpoint to fetch all users:
javascriptCopy codeapp.get('/api/users', async (req, res) => {
try {
const db = client.db('mydatabase');
const users = await db.collection('users').find().toArray();
res.status(200).json(users);
} catch (error) {
res.status(500).send({ error: 'Failed to fetch users' });
}
});
This code queries the users
collection and returns all documents. It’s a simple yet powerful way to access your MongoDB data.
4. Update Operation (Modify Data)
Updating existing records is a common requirement. Let’s add a PUT endpoint to update a user’s information based on their ID.
javascriptCopy codeapp.put('/api/users/:id', async (req, res) => {
const { id } = req.params;
const updatedUser = req.body;
try {
const db = client.db('mydatabase');
const result = await db.collection('users').updateOne({ _id: new ObjectId(id) }, { $set: updatedUser });
res.status(200).send(result);
} catch (error) {
res.status(500).send({ error: 'Failed to update user' });
}
});
This endpoint finds a user by their unique ID and updates their information. Using $set
ensures only the specified fields are modified without affecting the entire document.
“The ability to manipulate data directly from your application backend is what sets a robust API apart from a static one.”
5. Delete Operation (Remove Data)
Finally, the Delete operation allows you to remove documents from the collection. Let’s implement a DELETE endpoint.
javascriptCopy codeapp.delete('/api/users/:id', async (req, res) => {
const { id } = req.params;
try {
const db = client.db('mydatabase');
const result = await db.collection('users').deleteOne({ _id: new ObjectId(id) });
res.status(200).send(result);
} catch (error) {
res.status(500).send({ error: 'Failed to delete user' });
}
});
This endpoint deletes a user document by its ID. It’s useful for scenarios where users can manage their accounts and remove their data.
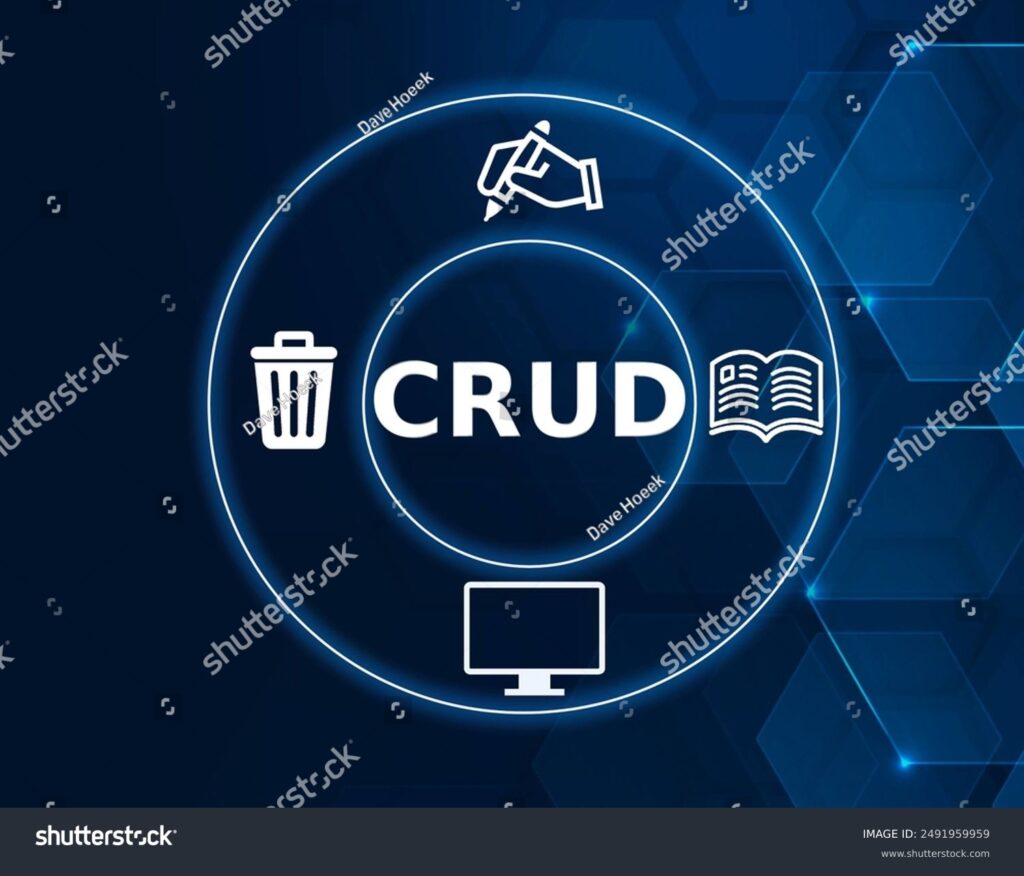
6. Error Handling and Best Practices
Handling errors gracefully is a key part of building reliable applications. Here are some best practices:
- Use Try-Catch Blocks: Wrap your database operations in try-catch blocks to catch and handle errors effectively.
- Validate User Input: Always validate the input data before inserting or updating records in the database.
- Close Database Connections: Ensure that database connections are properly closed when your application shuts down.
7. Conclusion
By mastering CRUD operations with MongoDB and Node.js, you unlock the potential to build scalable and efficient backend systems. Whether you’re creating a simple API or a complex application, understanding how to manipulate data is a fundamental skill for any developer.
For a deeper dive into backend development and integrating MongoDB with Express, explore this comprehensive guide. Also, if you’re curious about how Node.js can be used across various projects, this introduction to Node.js is a great resource.
With the right approach and tools, you can streamline your development process and deliver robust applications that meet the demands of modern users.