APIs (Application Programming Interfaces) play a vital role in modern web development, allowing different software applications to communicate and exchange data. With the rise of dynamic, data-driven websites, understanding how to effectively use APIs in JavaScript is an essential skill for developers. In this guide, we will explore what APIs are, how to work with the Fetch API, and how to handle API responses seamlessly using modern JavaScript features.
Table of Contents
- What Are APIs and How Do They Work?
- Introduction to the Fetch API
- Using Fetch for GET Requests
- Handling API Responses
- POST Requests with Fetch
- Error Handling in Fetch
- Real-World Examples of API Integration
- Best Practices for Working with APIs
- Conclusion
1. What Are APIs and How Do They Work?
An API is a set of rules and protocols that allows software applications to interact with each other. APIs act as intermediaries, enabling the transfer of data between systems without exposing internal implementation details. Whether you’re pulling weather data from an external service or posting form data to a server, APIs are your go-to tool for seamless integration.
“APIs are the building blocks of modern software applications, enabling connectivity and data exchange like never before.” — Linus Torvalds
To understand the power of APIs in JavaScript, let’s dive into the Fetch API—a modern and flexible way to make network requests.
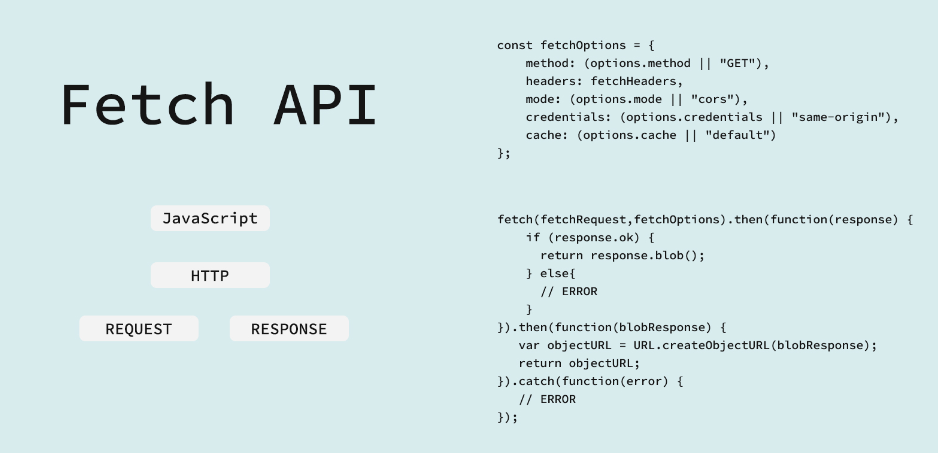
2. Introduction to the Fetch API
The Fetch API is a built-in JavaScript interface that allows you to make HTTP requests and handle responses. It offers a more powerful and flexible alternative to the older XMLHttpRequest
method. With Fetch, you can easily make GET, POST, PUT, and DELETE requests to interact with APIs.
Check out this resource for a deeper dive into JavaScript fundamentals: Introduction to ES6 JavaScript Features.
3. Using Fetch for GET Requests
A GET request is used to retrieve data from an API endpoint. Here’s a simple example using Fetch:
javascriptCopy codefetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => console.error('Error:', error));
In this code:
fetch()
initiates a network request to the specified URL.response.json()
parses the response data as JSON.- The data is logged to the console if the request is successful.
- Errors are handled gracefully using
.catch()
.
For a comparison between REST APIs and GraphQL APIs, refer to this post: GraphQL vs REST: Why Modern APIs Are Embracing Flexibility Over Tradition.
4. Handling API Responses
When working with APIs, handling responses correctly is crucial. Fetch returns a Response
object, which has various methods for processing the data:
text()
: Returns the response as plain text.json()
: Parses the response as JSON.blob()
: Returns the response as a binary large object (useful for file downloads).
Example:
javascriptCopy codefetch('https://api.example.com/user')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json();
})
.then(userData => console.log(userData))
.catch(error => console.error('Fetch error:', error));
“Error handling in API requests is as essential as the request itself; it ensures your application can gracefully recover from unexpected issues.” — John Resig
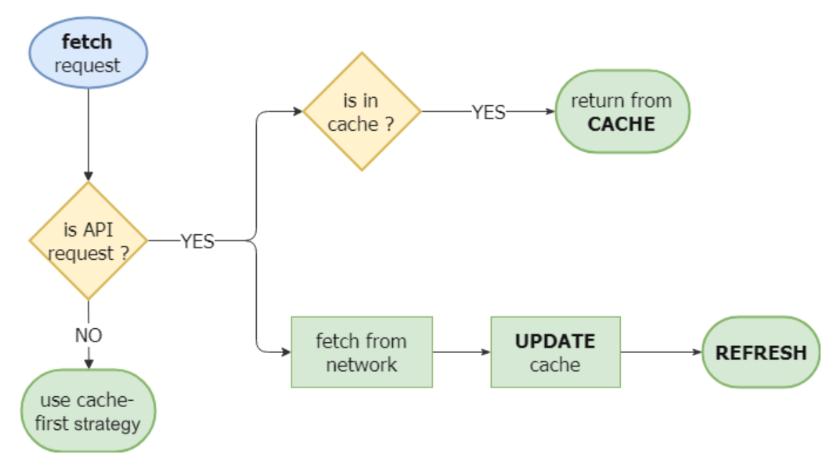
5. POST Requests with Fetch
A POST request is used to send data to an API. Here’s how you can send a POST request using Fetch:
javascriptCopy codeconst postData = {
name: 'John Doe',
email: '[email protected]'
};
fetch('https://api.example.com/create', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(postData)
})
.then(response => response.json())
.then(data => console.log('Success:', data))
.catch(error => console.error('Error:', error));
In this example:
- The
method
is set toPOST
. headers
define the content type.body
contains the JSON data to be sent.
This method is efficient when you need to submit forms or send structured data.
6. Error Handling in Fetch
Proper error handling ensures that your application can handle network issues gracefully. Use .catch()
for network errors and response.ok
to check for HTTP response status codes.
javascriptCopy codefetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => alert('There was a problem with your request: ' + error.message));
7. Real-World Examples of API Integration
In real-world scenarios, you may need to integrate APIs for tasks like:
- Fetching user information from a database.
- Displaying live data (e.g., weather updates).
- Submitting user-generated content (e.g., comments, posts).
For more on handling events in JavaScript, check this guide: Event Handling in JavaScript.
8. Best Practices for Working with APIs
- Use Async/Await: Modern JavaScript supports async/await, making code more readable.
- Validate API Responses: Always check the response status and handle errors properly.
- Limit API Requests: Avoid making excessive requests to prevent rate-limiting by the API provider.
Example using async/await:
javascriptCopy codeasync function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
if (!response.ok) throw new Error(`Error: ${response.status}`);
const data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
}
fetchData();
“Using async/await in JavaScript not only improves code readability but also simplifies asynchronous operations.” — Douglas Crockford
9. Conclusion
Mastering APIs and Fetch in JavaScript is crucial for building dynamic, data-driven applications. Whether you’re working with simple REST APIs or integrating complex third-party services, understanding these concepts will enhance your development skills.
For more advanced topics and a deeper dive into JavaScript, explore this comprehensive guide: Introduction to ES6+ JavaScript Features.