Middleware functions are a powerful feature of Express.js that enables developers to handle and manipulate HTTP requests before they reach the final route handler. By leveraging middleware, you can extend your app’s functionality, handle errors, manage authentication, and more. In this guide, we’ll dive into middleware in Express.js and explore how it can enhance the capabilities of your applications.
What is Middleware in Express.js?
Middleware in Express.js is essentially a function that has access to the request, response, and next objects in an application’s request-response cycle. Middleware functions can modify request and response objects, terminate the request-response cycle, or call the next function in the stack. This modular approach makes it easier to apply reusable functionality across routes.
“Middleware functions act as the bridge between requests and responses, enabling custom transformations, checks, and flows in Express applications.”
Middleware functions come in different types, including application-level, router-level, error-handling, and built-in middleware provided by Express.
Basic Setup for Middleware
To set up middleware in Express.js, you can define a function that processes requests and use app.use()
to apply it at the application level.
Here’s a simple example of setting up a middleware function to log request details:
javascriptCopy codeconst express = require('express');
const app = express();
app.use((req, res, next) => {
console.log(`Request Method: ${req.method}, Request URL: ${req.url}`);
next();
});
app.get('/', (req, res) => {
res.send('Welcome to the homepage!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
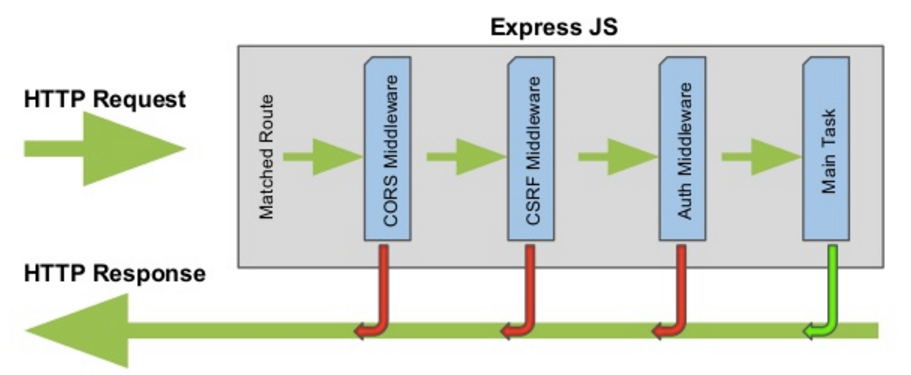
Common Use Cases for Middleware in Express.js
- Logging: Middleware can log requests for monitoring purposes.
- Authentication: Middleware can check for user authentication tokens.
- Error Handling: Middleware functions handle errors consistently across your application.
- Performance Optimization: Middleware can manage tasks like caching to enhance application performance.
For more on optimizing full-stack applications, consider exploring this practical guide to performance optimization, which offers insights that apply to both frontend and backend frameworks like Express.
Built-in Middleware in Express.js
Express.js provides a few built-in middleware functions to manage common tasks. For example:
- express.json(): Parses incoming JSON requests.
- express.static(): Serves static files such as images, CSS, and JavaScript.
Using these built-in functions can simplify your development, especially when paired with an understanding of JavaScript fundamentals. If you’re new to JavaScript, our Introduction to JavaScript Syntax and Fundamentals article offers an excellent starting point.
Custom Middleware in Express.js
Creating custom middleware functions is straightforward in Express.js. Custom middleware can be used to add any specific functionality your application requires.
For example, here’s how you might create middleware to check if a user is authenticated before accessing certain routes:
javascriptCopy codeconst isAuthenticated = (req, res, next) => {
if (req.headers.authorization) {
next();
} else {
res.status(403).send('Unauthorized');
}
};
app.get('/dashboard', isAuthenticated, (req, res) => {
res.send('Welcome to your dashboard!');
});
In this example, the isAuthenticated
middleware checks for an authorization header before allowing access to the /dashboard
route.
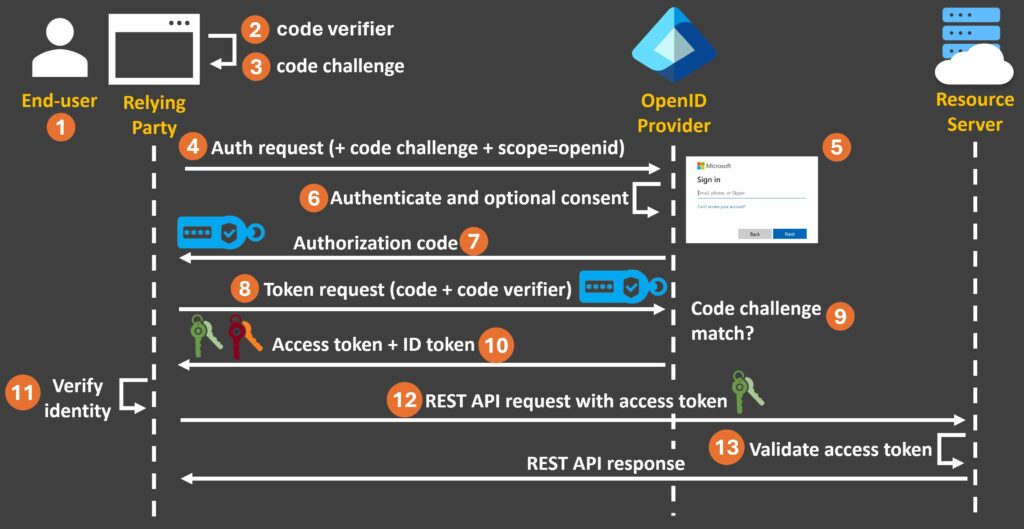
Enhancing Middleware with CSS Frameworks
Express middleware can also serve static files, enabling you to incorporate CSS frameworks into your application for a more polished frontend. CSS frameworks like Bootstrap simplify styling, allowing you to focus on the backend logic without sacrificing design quality. You can learn more about using CSS frameworks effectively in our guide, Introduction to CSS Frameworks (e.g., Bootstrap).
Conclusion
Middleware is an essential feature of Express.js that allows you to implement modular functionality, enhance security, and streamline error handling in your applications. From logging and authentication to serving static files and handling errors, middleware functions play a critical role in maintaining a clean and organized Express application structure.
As you work with middleware, keep refining your approach to improve both security and performance. Mastering middleware not only elevates your backend development skills but also helps in building efficient, high-performing applications.