In the world of relational databases, PostgreSQL and MySQL stand out as two of the most popular choices for developers and businesses alike. Both are powerful, open-source databases that can handle vast amounts of data efficiently. Whether you’re building a small web application or a large enterprise system, understanding the differences and how to work with both databases is crucial.
In this blog post, we will explore the features of PostgreSQL and MySQL, discuss how they differ, and provide a step-by-step guide on how to set up and perform basic operations with each. By the end of this guide, you’ll have a solid understanding of these two databases and how to choose the right one for your project.
1. Introduction to Relational Databases
Before diving into PostgreSQL and MySQL, it’s essential to understand the concept of relational databases. A relational database organizes data into tables (relations), where each table consists of rows and columns. This structured approach ensures data integrity and allows for complex querying.
For a deeper dive into the basics of relational databases and a comparison between SQL and NoSQL options, refer to this comprehensive introduction to database management.
2. What is PostgreSQL?
PostgreSQL, often referred to as Postgres, is an advanced open-source relational database management system (RDBMS). It is known for its support of complex queries, ACID (Atomicity, Consistency, Isolation, Durability) compliance, and extensibility. PostgreSQL is often favored for applications requiring complex data operations and strong data integrity.
Key Features of PostgreSQL:
- Support for Advanced Data Types: PostgreSQL supports JSON, arrays, and even custom data types.
- Extensibility: Allows users to define custom functions, operators, and data types.
- Strong ACID Compliance: Ensures reliable transactions and data integrity.
Let’s set up PostgreSQL and connect it to a Node.js application.
Setting Up PostgreSQL:
- Download and install PostgreSQL from the official website.
- Initialize the database using the
psql
command-line tool. - Create a database and user:
sqlCopy codeCREATE DATABASE mydatabase;
CREATE USER myuser WITH ENCRYPTED PASSWORD 'mypassword';
GRANT ALL PRIVILEGES ON DATABASE mydatabase TO myuser;
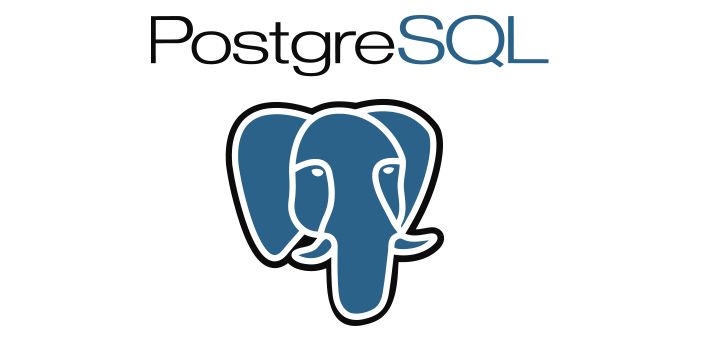
3. What is MySQL?
MySQL is another widely-used open-source relational database. It is known for its speed, reliability, and ease of use. MySQL is often the preferred choice for web applications and content management systems like WordPress due to its simplicity and strong community support.
Key Features of MySQL:
- Fast and Efficient: Optimized for read-heavy operations and fast data retrieval.
- Wide Adoption: Supported by a vast ecosystem of tools and frameworks.
- Ease of Use: Simple to set up and use, making it a great choice for beginners.
Setting Up MySQL:
- Download and install MySQL from the official website.
- Initialize the MySQL server and secure the installation.
- Create a database and user:
sqlCopy codeCREATE DATABASE mydatabase;
CREATE USER 'myuser'@'localhost' IDENTIFIED BY 'mypassword';
GRANT ALL PRIVILEGES ON mydatabase.* TO 'myuser'@'localhost';
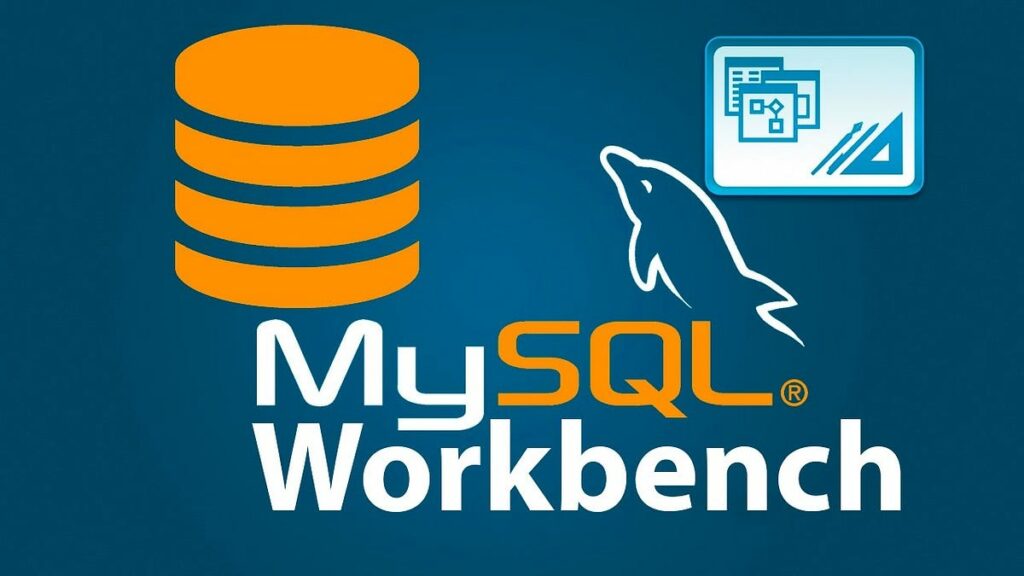
4. PostgreSQL vs. MySQL: Key Differences
While both PostgreSQL and MySQL are relational databases, they have distinct differences that might influence your choice depending on your project requirements.
Feature | PostgreSQL | MySQL |
---|---|---|
Complex Queries | Supports advanced queries and joins | Simple queries and basic joins |
Data Integrity | Strong ACID compliance | ACID compliance, but slightly weaker |
Performance | Optimized for write-heavy tasks | Optimized for read-heavy tasks |
Extensibility | Highly extensible | Limited extensibility |
For a deeper understanding of how relational databases differ from non-relational options like MongoDB, check out this guide on using MongoDB with Node.js.
5. Performing CRUD Operations with PostgreSQL and MySQL
CRUD operations (Create, Read, Update, Delete) form the backbone of any database-driven application. Let’s look at how to perform these operations with PostgreSQL and MySQL using Node.js.
5.1. Create Operation (Inserting Data)
PostgreSQL:
javascriptCopy codeconst { Client } = require('pg');
const client = new Client({
user: 'myuser',
host: 'localhost',
database: 'mydatabase',
password: 'mypassword',
port: 5432,
});
client.connect();
client.query("INSERT INTO users (name, email) VALUES ('John Doe', '[email protected]')", (err, res) => {
if (err) throw err;
console.log('User added:', res);
});
MySQL:
javascriptCopy codeconst mysql = require('mysql2');
const connection = mysql.createConnection({
host: 'localhost',
user: 'myuser',
password: 'mypassword',
database: 'mydatabase',
});
connection.query("INSERT INTO users (name, email) VALUES ('Jane Doe', '[email protected]')", (err, results) => {
if (err) throw err;
console.log('User added:', results);
});
6. Best Practices for Working with Relational Databases
- Normalize Your Database: Avoid data redundancy by normalizing your tables.
- Use Prepared Statements: Protect your application from SQL injection by using parameterized queries.
- Regular Backups: Ensure data safety with regular backups of your database.
If you’re developing a modern JavaScript application, you might find it helpful to review the key features of ES6 for writing cleaner, more efficient code.
“A well-structured database is the backbone of any efficient and scalable application.”
7. Conclusion
Both PostgreSQL and MySQL are excellent choices for relational databases, each offering unique features and benefits. PostgreSQL shines in scenarios requiring complex queries and strong data integrity, while MySQL excels in speed and simplicity. Understanding their strengths and how to implement CRUD operations with them will help you make informed decisions when building your next project.
If you’re still unsure which database to choose or want a broader understanding of database management, check out this detailed introduction to database management. Additionally, if you’re working on integrating MongoDB as well, our guide on using MongoDB with Node.js offers insights into working with NoSQL databases.
By mastering PostgreSQL and MySQL, you equip yourself with versatile skills that are crucial in today’s development landscape.