React has become a cornerstone of modern front-end development, empowering developers to create fast, dynamic, and interactive user interfaces. This powerful library, originally developed by Facebook, introduces a new way of thinking about building UI with components and JSX (JavaScript XML). In this comprehensive guide, we’ll dive deep into React components, the core building blocks of React applications, and JSX, the syntax extension that makes writing React code easier and more expressive.
What Are React Components?
React components are the building blocks of any React application. They help divide the UI into smaller, reusable parts, making it easier to manage and maintain. Components can be classified into two main types:
- Functional Components: These are simple JavaScript functions that take props as input and return React elements. They are stateless by design and have gained popularity with the introduction of React Hooks, which enable them to manage state.
- Class Components: Previously the standard way of writing components, class components are more feature-rich, supporting state and lifecycle methods directly within the class. However, with React Hooks becoming mainstream, functional components are now preferred for most use cases.
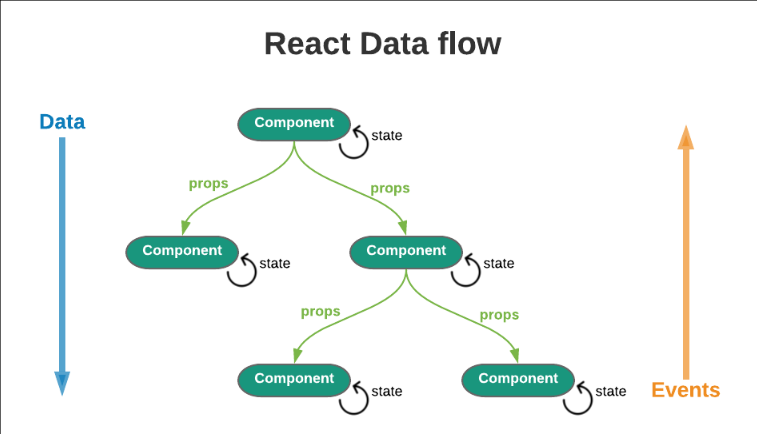
Understanding JSX: Writing HTML in JavaScript
JSX, or JavaScript XML, is a syntax extension for JavaScript that allows developers to write HTML-like code directly in their JavaScript files. While it looks like HTML, it’s actually transformed into React elements under the hood.
Example:
jsxCopy codefunction WelcomeMessage() {
return <h1>Welcome to React!</h1>;
}
This JSX code compiles to:
jsxCopy codeReact.createElement('h1', null, 'Welcome to React!');
JSX is not mandatory in React, but it simplifies code, making it more readable and intuitive.
Breaking Down a React Component
Let’s create a simple functional component to understand how components work.
Example:
jsxCopy codefunction Greeting({ name }) {
return <h2>Hello, {name}!</h2>;
}
In this example:
Greeting
is a functional component that takesprops
(in this case, aname
).- It returns a JSX element (
<h2>
), which is rendered on the screen.
You can use this component in your main application file like so:
jsxCopy code<Greeting name="Alice" />
The Power of Reusable Components
One of the biggest advantages of React is its ability to create reusable components. For instance, a button component can be reused across multiple pages, reducing redundancy and making your code DRY (Don’t Repeat Yourself).
“The beauty of React lies in its component-based architecture, allowing for the creation of reusable and maintainable user interfaces.” — Dan Abramov
Working with React Props
Props (short for properties) are the inputs to React components. They are used to pass data from parent components to child components.
Example:
jsxCopy codefunction UserCard({ name, age }) {
return (
<div>
<h3>{name}</h3>
<p>Age: {age}</p>
</div>
);
}
In this case, name
and age
are props passed to the UserCard
component:
jsxCopy code<UserCard name="John Doe" age={30} />
State in React Components
State is a special object that holds dynamic data in a React component. Unlike props, which are read-only, state can be changed over time, allowing components to respond to user actions.
To manage state in functional components, we use the useState Hook. For a deeper dive into state and props, check out React State and Props Management.
Example:
jsxCopy codeimport { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
In this example, useState
is used to create a piece of state called count
, which can be updated with the setCount
function.
Introducing React Hooks: Simplifying Functional Components
React Hooks like useState and useEffect have revolutionized the way we write React components. They allow functional components to manage state and handle lifecycle events, which was previously only possible with class components.
For a deeper understanding of React Hooks, refer to React Hooks: useState & useEffect.
JSX Best Practices
When working with JSX, following best practices can help you write more efficient and maintainable code:
- Use Self-Closing Tags: If an element has no children, use self-closing tags.jsxCopy code
<input type="text" placeholder="Enter your name" />
- Wrap Elements with Fragments: If a component returns multiple elements, wrap them in a React fragment (
<>...</>
).jsxCopy code<> <h1>Title</h1> <p>Description</p> </>
- Avoid Inline Styles: Instead of using inline styles, define a separate CSS file or use a styling library for consistency.
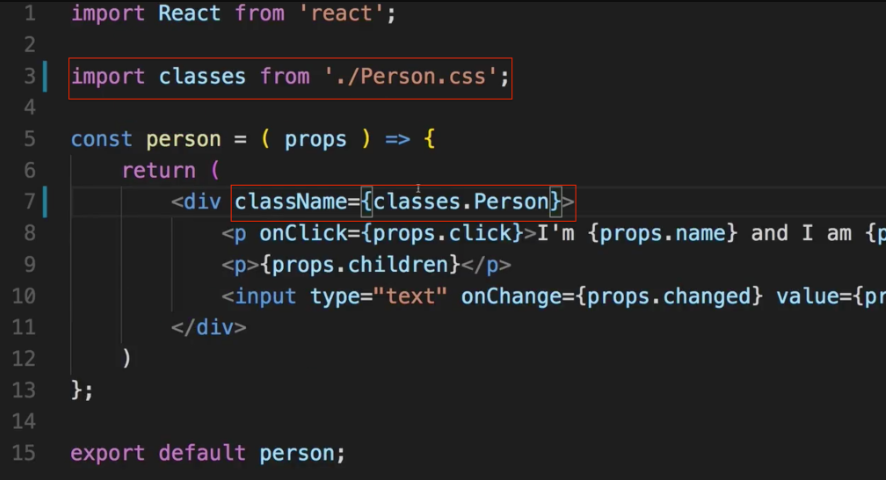
Handling Props and State Together
React components often use both props and state together for more complex UI interactions. Props provide the initial data, while state allows the component to handle changes and user interactions.
Example:
jsxCopy codefunction UserProfile({ initialName }) {
const [name, setName] = useState(initialName);
return (
<div>
<h2>User: {name}</h2>
<button onClick={() => setName('Updated Name')}>Change Name</button>
</div>
);
}
React’s component-based approach, combined with JSX, provides a powerful and intuitive way to build dynamic user interfaces. By understanding how to effectively use components, props, and state, you can harness the full potential of React in your projects.
“React’s declarative nature and component-driven approach have set a new standard for building scalable user interfaces.” — Kent C. Dodds
For more insights into JavaScript frameworks, explore this overview of JavaScript frameworks and libraries.